In this tutorial, we will take you through how to check if a file exists using Python.
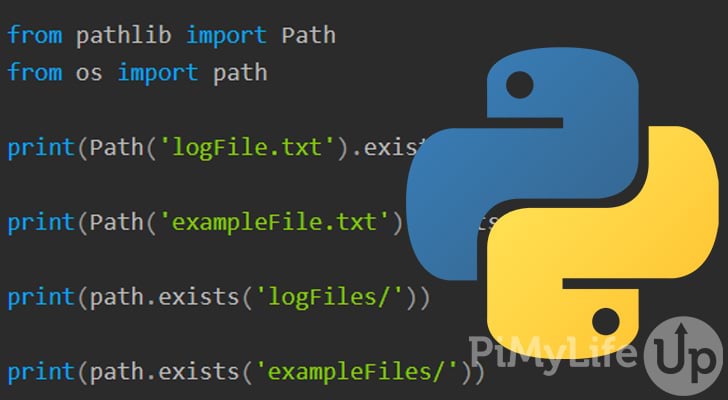
If you plan on using files in your Python script, you will likely want to check whether the file exists before performing any file operations. Luckily, checking if a file exists is straightforward in Python, thanks to the pathlib and os modules.
You can use Python to open, read, write, and close files. These operations will be instrumental if you do a lot of work with files, such as logs. As we mentioned above, it is good practice to check if the file exists before attempting to access a file.
In this tutorial, we will take you through several different methods of checking whether a file exists in Python. The first method uses the pathlib module, while the second uses the os module.
Using pathlib.Path to Check if a File Exists
From Python 3.4 onwards, the pathlib module is available in your Python scripts. The pathlib module allows you to perform a whole range of tasks involving file system paths on different operating systems. For this tutorial, we will be focusing on the exists method.
Below we will go through a small Python script demonstrating how the pathlib module works.
First, we import the main class named Path from the pathlib module.
Next, we have a print function that contains a path query. The path query has our file name as a parameter, and our file is located in the current working directory. Lastly, we want to use the exists()
method to determine if the directory or file exists.
You can use either absolute or relative paths as the parameter in Path. An absolute path starts from the root directory and a relative path starts from the current working directory.
Lastly, we repeat the same as above, but our exampleFile.txt
file does not exist.
from pathlib import Path
print(Path('logFile.txt').exists())
print(Path('exampleFile.txt').exists())
Our result below shows that our logFile.txt
exists, but the exampleFile.txt
does not exist.
python fileExists.py
True
False
If your file path points to a symlink, the result will be based on whether the symlink points to an existing directory or file.
Using os.path to Check if a File Exists
Our second method will make use of the os module in Python. The os module contains a range of tools for utilizing operating system dependent functionality. For example, to check if a file exists, we will be using the os.path
module.
As a parameter in os.path
you can use either absolute or relative paths. An absolute path starts from the root directory and a relative path starts from the current working directory.
os.path.exists()
The os.path.exists(path)
method will return true if the path exists. It will return false if the path does not exist or is a broken symbolic link. It may also return false if permissions are not granted to execute os.stat()
.
Below is an example of using this method in your Python code.
Firstly, we import our path module from the os module.
Next, we have a print function that contains path.exists()
. The path.exists()
method has a string containing our path as the parameter.
Lastly, we repeat the same as above, but our second path does not exist.
from os import path
print(path.exists('logFiles/'))
print(path.exists('exampleFiles/'))
As expected, our first print outputs true as the path exists, but our second print outputs false as the path does not exist.
python fileExists.py
True
False
os.path.isfile()
By using os.path.isfile(path)
we can check if our path is a regular file. It will follow symbolic links, so it will return true if the link points to a file.
The syntax of isfile()
is the same as exists()
and accepts the path as a parameter. You will need to import the path
module from the os module.
The example below has two different examples of the isfile()
method. The first method has an existing file as the path. The second method has a directory that also exists.
from os import path
print(path.isfile('logfile.txt'))
print(path.isfile('logFiles/'))
The first print statement outputs true as the path leads to an existing file. However, second print statement will return false as it points to a directory, not a file.
python fileExists.py
True
False
os.path.isdir()
You can use the os.path.isdir(path)
method to check if the path is a directory. It will follow symbolic links, so it will return true if the link points to a directory.
The isdir()
method accepts a path as a parameter. You will need to import the path module from the os module to use it.
The example below has two examples of using the isdir()
method. The first isdir()
method contains a path to a directory, so it will return true. The second isdir()
method contains a file, so it will return false.
from os import path
print(path.isdir('logFiles/'))
print(path.isdir('logfile.txt'))
As you can see below, our first print function outputs true as the path is a directory. However, the second print function outputs false as the path is a file, not a directory.
python fileExists.py
True
False
Conclusion
I hope by now you have a decent understanding of how you can check if a file exists in Python. Checking if a file exists is very beneficial if your Python script uses files. For example, you may want to create a file or stop the script from running if a required file is missing.
We have plenty of other Python tutorials that might interest you. For example, we have tutorials covering logical operators, converting string to an int, and lots more.
Please let us know if you notice a mistake or if an important topic is missing from this guide.