In this tutorial, we will take you through each of the arithmetic operators that you can use in Python.
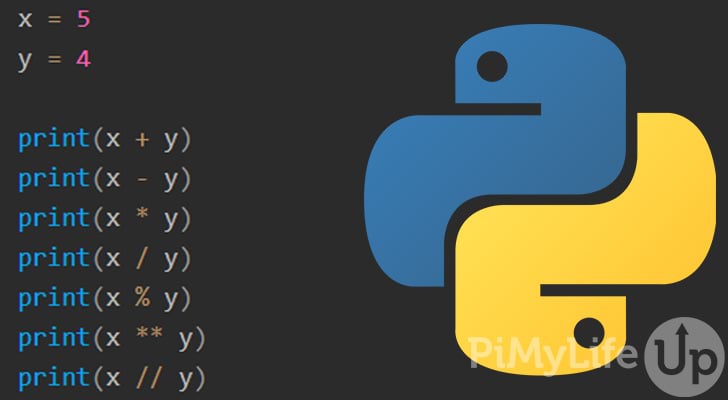
You will likely need to use Python’s arithmetic operators as they allow you to perform addition, subtraction, multiplication, division, modulus, exponent, and floor division on two or more values.
Below is a table that goes through each arithmetic operator you can use within Python. Further down the page, we go through each operator in more detail. However, the table is an excellent quick reference guide.
Operator | Description | Example |
---|---|---|
+ | Addition – adds two values together | x + y |
– | Subtraction – minuses the right value from the left value. | x – y |
* | Multiplication – multiplies the left value with the right value. | x * y |
/ | Division – divides the left value by the right value. | x / y |
% | Modulus – divides the left value by the right value but only returns the remainder. | x % y |
** | Exponent – left value to the power of the right value. | x ** y |
// | Floor Division – divides the left value by the right value but returns the lower integer. | x // y |
This tutorial assumes you are using Python 3 or newer. If you are using an older version of Python, there may be slight syntax and terminology differences from what is described in this tutorial.
Example of Arithmetic Operators in Python
We have a few examples to help demonstrate how each arithmetic operator works in Python. Each example will take you through how to use the operator and the expected output.
In this tutorial, we will be referring to operands quite a bit. Operands are the variables being evaluated by the operation. For example, the x
and y
in the following expression are the operands, and the +
is the operator.
x + y
Addition (+) Operator
The addition operator is represented by the plus +
symbol and allows you to add two values together.
In our example below, we add our x
and y
values together, then print the result on the terminal.
x = 5
y = 4
print(x + y)
Copy
As you would expect, 5 + 4
will output the value of 9
.
9
Subtraction (-) Operator
You can use the subtraction operator to subtract the second operand from the first operand. The subtraction operator is represented by the minus -
symbol.
The example below minuses the value of y
from the value of x
. The result of our calculation is printed to the terminal.
x = 5
y = 4
print(x - y)
Copy
The output printed to the terminal will be 1
as our math equation is the following 5 - 4
.
1
Multiplication (*) Operator
The multiplication operator is represented by the *
symbol and allows you to multiply the x
value by the value of y
.
Our example below multiplies the value of x
by the value of y
. The result of our multiplication is outputted to the terminal.
x = 5
y = 4
print(x * y)
Copy
Our mathematical equation is 5 * 4
, so the resulting output will be 20
.
20
Division (/) Operator
You will need to use the /
symbol to use the division operator. Using this operator will allow you to divide one value by another. In our case, divide value x
by value y
.
The example below will divide our x
value by the y
value. The print function will print the answer to our math problem to the terminal.
x = 5
y = 4
print(x / y)
Copy
The output printed to the terminal will be 1.25
as our math equation is 5 / 4
.
1.25
Modulus (%) Operator
The modulus operator will return the remainder of the division of two numbers. The symbol you can use to perform a modulo operation is the %
sign.
The best way to think of this math equation is in terms of a pizza. For example, if you have 5
slices of pizza and take 4
, you are left with 1
. Another example is if you have 10
slices of pizza and take 8
, you will be left with 2
.
We will use the modulus operator in our example below on our x
and y
values. The modulus operator means the result will be the remainder after dividing x
with y
.
x = 10
y = 4
print(x % y)
Copy
Our output is 2
as the remainder of 10
divided by 4
is 2
.
2
Exponentiation (**) Operator
The exponentiation operator will perform a “power to” calculation on a value. You can use the **
symbols if you need to raise the first operand to the power of the second operand.
Our example below uses the exponentiation operator on x
and y
. This math equation is the equivalent of saying raise x
to the power of y
.
x = 10
y = 4
print(x ** y)
Copy
The math equation above translates to 10
to the power of 4
, which works out to be 10000
.
10000
Floor division (//) Operator
The //
symbols represent the floor division operator. This operator will perform a regular division operation with two operands. However, this operator will return the largest possible integer. The integer will round down, so it will be equal to or less than the regular division result. For example, a division that equals 2.75
will be 2
.
The example below performs a floor division on the x
and y
values.
x = 11
y = 4
print(x // y)
Copy
The regular division of the above math equation equals 2.75
. However, since this is a floor division calculation, the result will be 2
.
2
Conclusion
I hope you now have a good understanding of each of the arithmetic operators that you can use in Python. You will find these operators extremely useful for performing mathematical equations. We touched on the following operators; addition, subtraction, multiplication, division, modulus, exponentiation, and floor division.
This tutorial only touches on arithmetic operators, but there are a ton of other operators that you can use within Python. For example, you will likely need to use logical or ternary operators.
Please let us know if you notice a mistake or if an important topic is missing from this guide.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support