In this tutorial, we will show you how you can check the version of Python running on your computer.
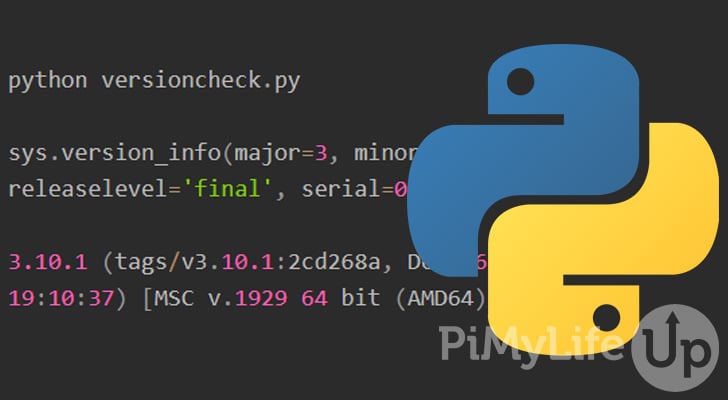
Python is a commonly used programming language that has received many updates over its lifetime, so you will likely need to check the version a system is running.
It is important to know the Python version running on your machine as each version has changes that may impact whether the code will behave how you expect it. The most significant changes usually occur between major updates. For example, going from Python 2 to Python 3.
The version number in Python is split into three numbers, 3.10.1
. 3 represents a major update, 10 is a minor update, and 1 is a micro update. Each update will also have a “release level” such as alpha, beta, candidate, and final. Final is the version you are most likely to use.
If you are on a Python version before Python 3.7, it is highly recommended that you update to the latest version, as older versions of Python might not get patched.
Check Python Version via Terminal
The easiest way to check the version of Python is to use the Python command in your machine’s terminal. If you do not have Python setup correctly, this method may not work, but you can check the version inside a script instead.
You can check the version of Python in the terminal by appending -V
or --version
after python
in the terminal. These are case-sensitive, so make sure you have the case correct; otherwise, you will see an error.
Below is an example of using the command.
python --version
Copy
Windows
Checking the version of Python on Windows is very straightforward, as shown in the steps below. If you haven’t already installed it, I recommend checking our guide on installing Python on the Windows operating system.
1. To get started, load the command prompt application by searching for “command prompt” or pressing WINDOWS KEY + R, then typing in CMD and pressing ENTER.
2. Within the command prompt, enter the following command.
python -V
Copy
3. If Python is installed correctly, you should see output similar to the example below appear in the command line.
C:\Users\gus>python -V
Python 3.10.1
Copy
macOS
Checking the Python version on macOS is similar to the other operating systems. Of course, you will need to install Python on macOS before proceeding with the following steps.
1. To check the version of Python installed on your macOS computer, you will need to open the terminal.
You can load the terminal by opening the launchpad and searching “terminal“. Click on Terminal.
Alternatively, you can go to finder and load the Applications folder. Next, find the Utilities folder. Then, inside utilities, click and load the terminal application.
2. In the terminal session, run the following command.
python -V
Copy
3. If Python is installed correctly, you will see an output similar to our example below appear in the command line.
Gus-MBP:~ gus$ python -V
Python 3.10.1
Copy
Linux
Checking the Python version in Linux is likely the easiest of the three. However, before proceeding with this section, you will want to ensure that you have Python installed on your Linux operating system.
1. To check the version of Python installed on your Linux system, you will need to be in the terminal. If you are using a desktop version of Linux, you may need to locate the terminal application.
For example, on the Ubuntu desktop, you can find the terminal application by clicking activities (top left corner) and searching terminal.
2. In the terminal session, run the following command.
python -V
Copy
3. If Python is installed correctly, you will see output like the example below appear in the command line.
dev@pimylifeup:~$ python3 -V
Python 3.8.10
Copy
Check the Python Version inside a Script
There are a couple of different methods that you can use to check the version of Python from within a Python script. We will touch on both of the methods below.
Using the sys Module
The sys module contains the Python version and additional information, such as the build and compiler.
In this example, we will request the version using two different functions, which I will quickly explain below.
- Using
sys.version
will return a string that contains the version number and a range of other pieces of information, such as the build number and compiler used. - Alternatively, you can use
sys.version_info
, which returns a tuple containing five pieces of info about a release. It will contain major, minor, micro, releaselevel, and serial. For example, Python 3.0 will be (3,0,0, ‘Final, 0).
In a Python script file, enter the following code.
import sys
print(sys.version_info)
print(sys.version)
Copy
If you run the above script in your computer’s terminal, you will get an output similar to the one below.
python versioncheck.py
sys.version_info(major=3, minor=10, micro=1, releaselevel='final', serial=0)
3.10.1 (tags/v3.10.1:2cd268a, Dec 6 2021, 19:10:37) [MSC v.1929 64 bit (AMD64)]
Copy
Using the platform Module
You can also use the platform module to get information on the current version of Python running on your machine. This module is similar to the sys module, but the function names are slightly different.
Below are a few examples of the functions you can use to access information.
- The
platform.python_version()
will return the version of Python. - Using
platform.python_compiler()
will return details about the compiler used to compile the build of Python you are using. - If you require information about the build, such as the date and build number, use the
platform.python_build()
function.
There are other functions that you can use, but these are the ones that will get you the information that you likely require.
In a Python script file, enter the following code.
import platform
print(platform.python_version())
print(platform.python_compiler())
print(platform.python_build())
Copy
Executing the script in your computer’s terminal will get an output similar to the one below.
Python versioncheck.py
3.10.1
MSC v.1929 64 bit (AMD64)
('tags/v3.10.1:2cd268a', 'Dec 6 2021 19:10:37')
Conclusion
I hope you now have a good understanding of how you can check the version of Python on each popular operating system and how you can check it in a Python script.
If you are new to Python, we recommend checking out some of our guides on effectively using the programming language. I recommend starting with our tutorial on getting started with Python.
If you have run into any issues or have any feedback, please feel free to drop a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support