In this tutorial, I go through the basics of Python tuples and how you can use one.
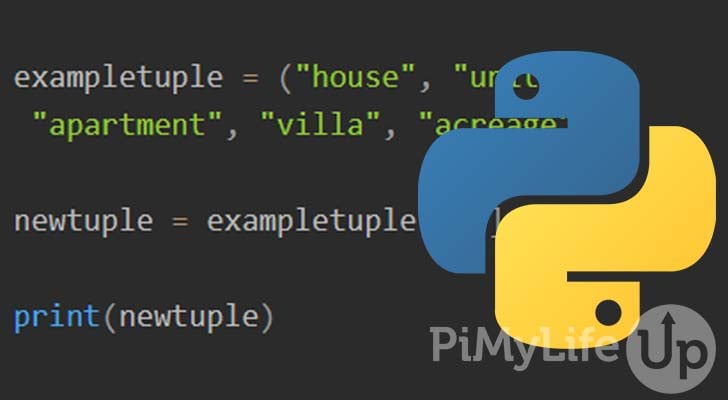
A tuple is a data type in Python used to store multiple items in a single variable. The items are ordered and are immutable after creation. The items stored in a tuple can be of any type.
The syntax of tuples and lists are similar. Tuples are typically enclosed by parentheses, but they are not required. Lists, on the other hand, are enclosed by square brackets.
It would be best to use a tuple whenever you have data that does not need to be altered after creation. Tuples are also faster than other data collection types, such as lists.
Table of Contents
- Creating a Tuple
- Accessing Values within a Tuple
- Updating Tuples
- Deleting Values within a Tuple
- Deleting a Tuple
- Tuple Built-in Functions
- Conclusion
Creating a Tuple
Creating a tuple in Python is super easy, but there are a few things that you will need to be aware of.
Tuples are typically enclosed by parentheses, but they are not required. Also, if you have just a single value in the tuple, it must be followed by a comma.
Below is an example of creating a tuple that contains three items.
exampletuple = ("house", "unit", "apartment")
If you use print to output the tuple into the terminal, you should get something similar to the following.
Python> python tuple.py
('house', 'unit', 'apartment')
A Tuple with a Single Item
If you want to create a tuple that contains just a single item, make sure you add a comma after the item. Python will not recognize it as a Tuple if you do not add the comma.
Below is a valid tuple within Python.
exampletuple = ("house",)
The example below is an invalid tuple in Python.
exampletuple = ("house")
Using the tuple() Constructor
You can also use the tuple constructor to create a valid tuple.
exampletuple = tuple(("house", "unit", "apartment"))
As you can see in our output below, it prints out the same value as creating the tuple without the constructor.
Python> python tuple.py
('house', 'unit', 'apartment')
Accessing Values within a Tuple
Accessing values inside a tuple is not much different from accessing a value in a list. In this section, we cover a few different techniques on how you can access the value you require.
Like an array, tuples in Python start counting from 0
, so to reference the first element, you will use an index value of 0
. With the index number, you can access values held by the tuple by using square brackets []
.
You can iterate through a tuple using a for loop or access the data directly by using an index number.
Index
In our example code, we create a tuple with 5 items. This number of items means the index will start at 0
and finish at 4
. Trying to access outside of this range will result in an error.
Lastly, we print out the values of indexes 0
and 3
of the tuple.
exampletuple = ("house", "unit", "apartment", "villa", "acreage")
print(exampletuple[0])
print(exampletuple[3])
Executing the code produces the following output. As you can see, index 0 is the first string, house. Also, item 3 is the fourth string, villa.
Python> python tuple.py
house
villa
Negative Index
You can use a negative index with a tuple data collection. Using negative numbers means the index of -1 refers to the last item and works backward the higher the negative number.
In our code below, we create a tuple with 5 items. We will access these items using a negative index instead of a positive.
exampletuple = ("house", "unit", "apartment", "villa", "acreage")
print(exampletuple[-1])
print(exampletuple[-3])
As you can see in our output below, we output the last item (-1) and the third item (-3) from our tuple.
Python> python tuple.py
acreage
apartment
Slicing
To access a range of values from a tuple, you can use the slicing operator :
. You specify the start and end of the range you want on each side of the colon. eg. [0:4]
will select everything between 0 and 4.
Slicing a tuple is slightly different as the numbers reference between elements rather than the element itself. Our diagram below demonstrates how the tuple ("P", "I", "M", "Y", "L", "I", "F", "E", "U", "P")
can be referenced when slicing.
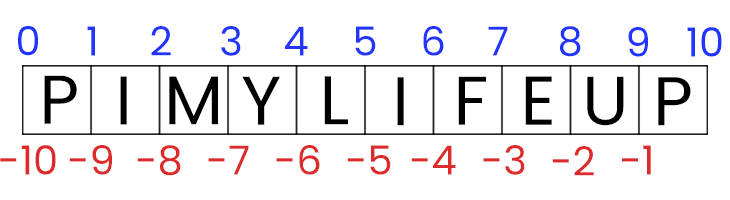
If you do not specify a number, it assumes either from the start or to the end. So, for example, [:3]
will be everything from element 0 to element 3. On the flip side, [1:]
is everything from element 1 to the end.
In our example below, we want everything between 1 and 3 of our example tuple.
exampletuple = ("house", "unit", "apartment", "villa", "acreage")
print(exampletuple[1:3])
You can see that our output has selected the elements between 1 and 3. You can adjust these numbers to select the data you need from the tuple.
Python> python tuple.py
('unit', 'apartment')
Updating Tuples
A tuple is immutable, so you cannot alter them once they have been created. However, you can create a new tuple from parts of an existing tuple.
exampletuple = ("house", "unit", "apartment", "villa", "acreage")
newtuple = exampletuple[0:3]
print(newtuple)
Our output below shows that our new tuple only contains part of our old tuple. You can go one step further and concatenate two tuples into a new tuple, and we cover this in the next section.
python tuple.py
('house', 'unit', 'apartment')
Concatenate Multiple Tuples
You may need to combine multiple tuples as a new tuple. For example, you want one part of a tuple to be merged into another tuple.
In our example below, we take a section of our original tuple and concatenate it with a new tuple. This concatenated tuple is stored in a new variable.
exampletuple = ("house", "unit", "apartment", "villa", "acreage")
newtuple = exampletuple[0:3] + ("ranch", "farm")
print(newtuple)
As you can see below, we successfully concatenated both of our tuples. Of course, it is just an example, and you can do this with more than two tuples.
python tuple.py
('house', 'unit', 'apartment', 'ranch', 'farm')
Deleting Values within a Tuple
As we mentioned before, tuples in Python are immutable, but it is still possible to delete values from a tuple using the methods mentioned in our previous topic.
In the example below, we remove the apartment element by slicing the tuple and leaving out that specific element.
exampletuple = ("house", "unit", "apartment", "villa", "acreage")
newtuple = exampletuple[:2] + exampletuple[3:]
print(newtuple)
In our output below, you can see that the apartment element has been successfully removed from the tuple. However, it does involve making a new tuple.
Python> python tuple.py
('house', 'unit', 'villa', 'acreage')
Deleting a Tuple
Deleting an entire tuple in Python is pretty straightforward and can be achieved using the del
statement. After deletion, any call to that tuple will result in an error unless you recreate it.
Below we create a tuple, print it, delete it and try printing it again.
exampletuple = ("house", "unit", "apartment")
print(exampletuple)
del exampletuple
print(exampletuple)
The output shows that Python deleted the tuple successfully as it resulted in an error when we tried printing it for a second time.
python tuple.py
('house', 'unit', 'apartment')
Traceback (most recent call last):
File "D:\Python\tuple.py", line 7, in <module>
print(exampletuple)
NameError: name 'exampletuple' is not defined
Tuple Built-in Functions
We have touched on some of the ways you can interact with a tuple in the above examples. However, below are a few more functions you can use with a tuple.
cmp(tuple1,tuple2) | Compares the elements of two tuples |
len(tuple) | Returns the total length of the tuple. |
all(tuple) | Returns true if all the tuple elements are true or the tuple is empty. |
any(tuple) | Returns true if any tuple element is true. Will return false if the tuple is empty. |
max(tuple) | Will return the maximum element of the tuple. |
min(tuple) | Returns the minimum element of the tuple. |
sum(tuple) | Sums all the numbers in the tuple. Results in an error if a string exists in the tuple. |
sorted(tuple) | Sorts the tuple. |
Conclusion
I hope that this guide has taught you all the basics of using tuples in Python. You should find tuples useful when coding complex Python programs, as there are benefits to using them over a list.
If you want to learn more about the Python programming language, I highly recommend checking out more of our tutorials. In addition, I recommend learning more about the collection data types that are available in Python. For example, lists, sets, and dictionaries all have their unique use cases in Python.
Please let us know if there are any improvements we can make to this tutorial by leaving a comment at the bottom of this page.