In this tutorial, I go through the basics of Python dictionaries and how you can use them.
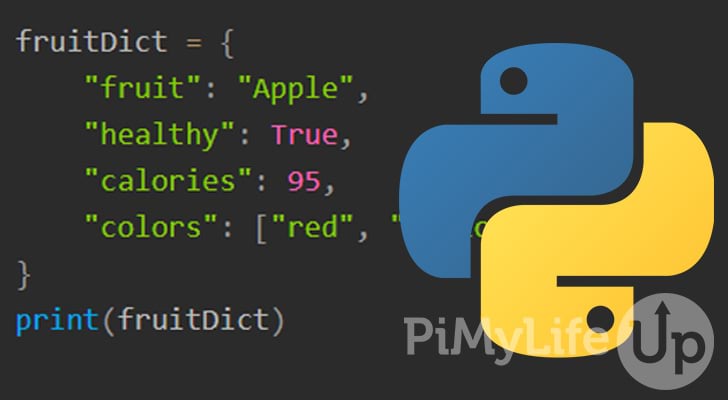
Dictionaries in Python are used to store multiple items within a single variable. Each item has a key and value, with the key being the identifier.
A dictionary is ordered and is mutable. So, being ordered means each item remains in order unless you change it, and mutable means you can make changes to the dictionary after creation.
The syntax of a dictionary is very straightforward. A dictionary contains a range of keys and values contained within curly brackets. Each key and value is separated by a colon. After every key and value pair, a coma signifies the end of the item.
It is best to use a dictionary when you need to associate values with a key. Using keys to access data can be more efficient, but it depends on the data you are handling. For example, you might want to use a list, tuple, or set instead.
This guide is written assuming that you are using Python 3 or later. Versions earlier than Python 3 may have some slightly different behaviors.
Table of Contents
- Creating a Dictionary
- Accessing Dictionary Items
- Adding Dictionary Items
- Updating Dictionary Items
- Deleting Dictionary Items
- Copying Dictionaries
- Delete a Dictionary
- Python Dictionary Built-in Methods
- Conclusion
Creating a Dictionary
Creating a Python dictionary is pretty easy, but there are a few things that you need to know. We will quickly go through some of the important features of a dictionary.
A dictionary is defined in Python by placing items within curly brackets. Each item in a dictionary consists of a key and a value. A colon separates the key and value. Each item should be on its own line and end with a comma. The following is an example of an item within a dictionary. "fruit": "Apple",
A dictionary is ordered; therefore, items will always be in the same place unless changed. In Python 3.6 and earlier, dictionaries were unordered.
Dictionaries are mutable so you can make changes to the values after creation. However, keys are immutable and must be removed and recreated if a change is required. We cover updating items and removing items further on in this tutorial.
You cannot have duplicate keys in a dictionary, so keep this in mind when planning your code. However, values can be duplicates.
Below are a couple of examples of creating a dictionary within Python.
Dictionary Example
Our example below demonstrates how to create a dictionary using curly braces. We also used mixed data types for each of the values. The keys are all strings, but can be integers.
fruitDict = {
"fruit": "Apple",
"healthy": True,
"calories": 95,
"colors": ["red", "yellow", "green"]
}
print(fruitDict)
Copy
Below is the output of our dictionary. We detail how to access specific values within a dictionary a bit further on in this tutorial.
python dictionary.py
{'fruit': 'Apple', 'healthy': True, 'calories': 95, 'colors': ['red', 'yellow', 'green']}
Copy
Using the Dictionary Constructor
You can create a dictionary using the built-in constructor function. Below are two methods on how you can use the constructor.
The first example is simply placing our dictionary syntax inside the dict()
function. The second example is a list of tuples as a parameter in the dict()
function.
fruitDict = dict({
"fruit": "Apple",
"color": "Red",
})
print(fruitDict)
fruitDict = dict([
("fruit", "Apple"),
("color", "Red"),
])
print(fruitDict)
Copy
Not surprisingly the output of both of the above examples is the same.
python dictionary.py
{'fruit': 'Apple', 'color': 'Red'}
{'fruit': 'Apple', 'color': 'Red'}
Nested Dictionary Example
There may be times where you want to create a nested dictionary, which is a dictionary within a dictionary. The example below demonstrates how you can nest a dictionary.
fruitDict = {
"fruit": "Apple",
"calories": {
"big":"130",
"medium":"95",
"small":"78"
}
}
print(fruitDict)
Copy
Below is the output from the above code. You can see all our keys, values, and nested dictionary.
python dictionary.py
{'fruit': 'Apple', 'calories': {'big': '130', 'medium': '95', 'small': '78'}}
Accessing Dictionary Items
Now that you have created your dictionary, you will likely want to know how to access the keys and values. There are a few different methods that we can use to access our data. We will touch on each of them below.
Using Keys
To access values associated with a key, you must specify the key using the get()
method or referencing it within square brackets.
If you specify a key that does not exist, you will get an error using the square brackets method. Alternatively, using the get()
method, the string “none” will be returned.
In the example below, we access the value associated with the fruit key by using the get()
method. The second print achieves the same thing but uses square brackets instead.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
print(fruitDict.get("fruit"))
print(fruitDict["fruit"])
Copy
As you would expect, the output is “Apple” both times.
python dictionary.py
Apple
Apple
Copy
Get All Keys
To get all the keys currently defined in a dictionary, you can use the keys()
method. Using keys()
creates a view of the dictionary, so our new variable will always reflect any changes made to the keys list in the dictionary.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitKeys = fruitDict.keys()
print(fruitKeys)
Copy
All the keys are returned within an iterable but non-indexable object. You can convert the object into a list using the list constructor if you wish to use an index, or you can use the object within a for loop if you want to iterate through the data.
python dictionary.py
dict_keys(['fruit', 'color', 'calories'])
Get All Values
You can access all the values within a list by using the values()
method. Using values()
will create a view to the dictionary, so any changes made to the dictionary will always be reflected in the values list.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitValues = fruitDict.values()
print(fruitValues)
Copy
All the values are returned within an iterable but non-indexable object. If you wish to access the data using an index, you will need to convert the object into a list by using the list constructor. Alternatively, you can iterate through the data using a for loop.
python dictionary.py
dict_values(['Apple', 'red', 95])
Check if a Key Exists
You can check that a key exists within a dictionary before proceeding with a task. All you need to do is use the in
keyword. Below is an example of an if conditional statement using the in
keyword.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
if "fruit" in fruitDict:
print("Yes, fruit exists within this dictionary.")
Copy
Since the fruit key exists in our dictionary, we get the print output when running the script.
python dictionary.py
Yes, fruit exists within this dictionary.
Adding Dictionary Items
There are a couple of ways to add new items to a dictionary.
Adding Items Using Square Bracket Notation
The first method is simply creating a new key and assigning a value to it. Below is an example of adding a new item using this method.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict["size"] = "small"
print(fruitDict)
Copy
The output of the script shows that our dictionary contains our new key and value.
python dictionary.py
{'fruit': 'Apple', 'color': 'red', 'calories': 95, 'size': 'small'}
Adding Items Using Update()
The second method uses the update method to create a new key and value. Typically, you will use this method to update existing keys, but it will create it if the key does not exist. The update method accepts a dictionary.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict.update({"size": "medium"})
print(fruitDict)
Copy
As you would expect, the output displays the dictionary with the new item.
python dictionary.py
{'fruit': 'Apple', 'color': 'red', 'calories': 95, 'size': 'medium'}
Updating Dictionary Items
Updating values within a dictionary is straightforward and can be done using two different methods.
Updating Items Using Square Bracket Notation
The first method, we update our value by referencing an existing key and assigning the new value to it. We update our fruit key from apple to orange in the example below.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict["fruit"] = "Orange"
print(fruitDict)
Copy
The output below shows that our fruit key now contains our updated value.
python dictionary.py
{'fruit': 'Orange', 'color': 'red', 'calories': 95}
Updating Items Using Update()
You can update multiple values at once by using the update()
method. You will need to supply a dictionary as a parameter to the update method, inside the dictionary simply reference the key and the value you wish to update.
Below is an example of updating every value held within our dictionary using the update method.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict.update({
"fruit": "Banana",
"color": "Yellow",
"calories": "105"
})
print(fruitDict)
Copy
You should see that all the values have been updated. Python will simply add a new entry into the dictionary if you misspell a key.
python dictionary.py
{'fruit': 'Banana', 'color': 'Yellow', 'calories': '105'}
Deleting Dictionary Items
You can use multiple methods to remove items from a dictionary. Each of these methods has its pros and cons, so ensure you understand each one before using them.
Using pop()
The pop()
method will remove a single key and its associated value. If you specify a key that does not exist, you will get a fatal error.
You will need to specify the key you wish to be removed as a parameter in the pop method.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict.pop("calories")
print(fruitDict)
Copy
In the output below, you can see that the specified key and its value have been deleted.
python dictionary.py
{'fruit': 'Apple', 'color': 'red'}
Using popitem()
You can use the popitem()
method to remove the last item in the dictionary. In Python versions before 3.7, popitem()
removed a random item.
There is no parameters required for popitem() to work.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict.popitem()
print(fruitDict)
Copy
The output below shows popitem()
removed the last item of our dictionary.
python dictionary.py
{'fruit': 'Apple', 'color': 'red'}
Using clear()
The clear method will remove every item from the dictionary. It is perfect if you want to start fresh.
There are no parameters that you need to supply to the clear method.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
fruitDict.clear()
print(fruitDict)
Copy
The output below shows that all the keys and their assigned values have been deleted.
python dictionary.py
{}
Using del
Another method of removing items from the dictionary is using the del keyword. To remove an item, write del
followed by the dictionary and key you wish to remove.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
del fruitDict["color"]
print(fruitDict)
Copy
In the output below, you can see that the specified key and its value have been deleted.
python dictionary.py
{'fruit': 'Apple', 'calories': 95}
Copying Dictionaries
You cannot copy directories by simply assigning them to a new variable, e.g., fruitDict2 = fruitDict
. By simply assigning a dictionary to a new variable, you create a reference to the original variable rather than making a copy.
There are two different techniques that you can use to copy a directory. Both will achieve the same task, but I prefer to use the copy()
method.
Using copy()
To copy a dictionary you can use the copy()
method.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
newDict = fruitDict.copy()
print(newDict)
Copy
As you can see our new dictionary is a copy of the old one. If you make changes to the copy, it will not affect the original.
python dictionary.py
{'fruit': 'Apple', 'color': 'red', 'calories': 95}
Using dict()
You can also create a copy of a dictionary by using the dict()
constructor. Simply use the dictionary as the parameter and dict()
will create a copy of it.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
newDict = dict(fruitDict)
print(newDict)
Copy
As you can see our new dictionary is a copy of the old one. If you make changes to the copy, it will not affect the original.
python dictionary.py
{'fruit': 'Apple', 'color': 'red', 'calories': 95}
Delete a Dictionary
Deleting an entire dictionary is pretty straightforward using the del
keyword. Simply write del
followed by the dictionary you wish to delete.
In the example below we print our dictionary, delete it, then attempt to print it again.
fruitDict = {
"fruit": "Apple",
"color": "red",
"calories": 95
}
print(fruitDict)
del fruitDict
print(fruitDict)
Copy
As you can see in the output below, the first print worked fine, but the second threw an error. The error occurred because we deleted the dictionary and tried to call a non-existent variable.
python dictionary.py
{'fruit': 'Apple', 'color': 'red', 'calories': 95}
Traceback (most recent call last):
File "D:\Python\dictionary.py", line 11, in <module>
print(fruitDict)
NameError: name 'fruitDict' is not defined
Python Dictionary Built-in Methods
There are quite a few different methods you can use on a Python dictionary. These methods will help you access data and make changes to the dictionary. You must understand each of these methods as it helps improve your code quite a bit.
Below are some of the built-in methods that you’re able to use.
dict.clear() | Clears the dictionary, so there are no items. This method doesn’t accept any parameters. |
dict.copy() | Returns a copy of the dictionary. This method doesn’t accept any parameters. |
dict.fromkeys(keys,val) | Returns a dictionary containing the specified keys and associated values. This method accepts two parameters. |
dict.get(key, val) | Will return the value of the specified key. Accepts two parameters; the second is optional. Key is the key you wish to search for. Val is the default value to display if no key is found. |
dict.items() | Returns a list that contains a tuple for each key pair. This method doesn’t accept any parameters. |
dict.keys() | Will return a list of all the keys within the dictionary. This method doesn’t accept any parameters. |
dict.pop(key, val) | Removes the element containing the specified key. This method accepts two parameters; the second is optional. Key is the key you wish to search for. Val is the default value to display if no key is found. |
dict.popitem() | Removes the last inserted key pair. This method doesn’t accept any parameters. |
dict.setdefault(key, default) | Attempts to fetch the value of the specified key. If the key does not exist, it inserts the key alongside the specified default value. |
dict.update(dict1) | Updates the dictionary with the specified key and value. This method accepts a dictionary as a parameter. |
dict.values() | Returns all the current values within the dictionary. This method doesn’t accept any parameters. |
Conclusion
I hope by now you have a good understanding of how to use dictionaries in Python. We have covered the basics, such as creating and deleting dictionaries. We also touched on updating and adding new items within a dictionary. All this information should help you create better code.
There is a lot more to learn about coding in Python, so I recommend checking out more of our tutorials. For example, if you want to learn more about the available collection types, I recommend checking out tutorials on lists, sets, and tuples.
Please let us know if there are any improvements we can make to this tutorial by leaving a comment at the bottom of this page.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support