In this Python tutorial, we will be explaining the Python programming language’s syntax.
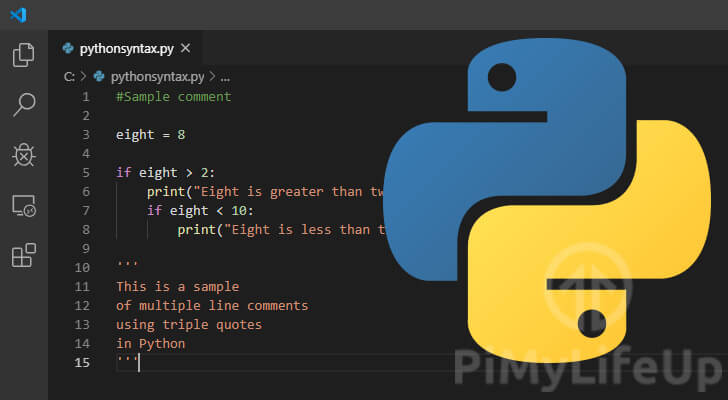
The syntax of a programming language is like the set of rules that define how your code should be written.
By gaining an understanding of Python’s syntax, you will be able to write code faster and easier while avoiding running into syntax errors.
One of the biggest things that you will need to learn to be able to write Python code is proper indentation.
Indentation in Python
Indentation is an incredibly important part of the Python programming language as it is used to define a code block.
Unlike most programming languages that use indentation purely for readability, Python makes use of whitespace (Spaces and Tabs) to define a code block.
Most other programming languages like C and C++ make use of braces ( { }
) to indicate blocks of code and is not dependent on the amount of white space.
There is some advantages to using indentation to define code blocks.
One of those advantages is that it forces you to have to write cleaner code. Missing indentation will break the script.
To define a code block in Python, you must have at least one space.
Example of Indentation
Below we have an example of a valid block of code in Python.
if 8 > 2:
print("Eight is greater than two!")
Copy
Forgetting to Indent
If you forget to indent the code, like below, the Python interpreter will throw a invalid syntax
error.
if 8 > 2:
print("Eight is greater than two!")
Copy
Make sure you always remember to indent your code blocks.
Different Whitespace in Different Code Blocks
The amount of whitespace you use in your Python code blocks is up to you. As long as each line of code in the block uses the same amount of whitespace.
Below is an example of two valid code blocks that have differing levels of whitespace.
if 8 > 2:
print("Eight is greater than two!")
print("This is fine as we are indented the same!")
if 8 > 2:
print("Eight is greater than two!")
print("This is fine as we are indented the same!")
Copy
While you can use different amounts of whitespace for different code blocks, we recommended you stick with a certain amount of whitespace per indent.
Keeping the same amount of whitespace for each code block helps you keep your code easily readable.
We recommend that you stick to about 4 spaces for each indent.
Different Whitespace in the Same Code Block
If you use different levels of whitespace within the same code block like below, you will run into a syntax error.
The reason for this is that Python will not be able to understand if the third line belongs within that code block or not.
if 8 > 2:
print("Eight is greater than two!")
print("This will throw a error as the indent differs!")
Copy
Make sure you always use the same amount of whitespace when indenting within the same block of code.
Indenting Nested Code Blocks
Below we have included an example of how two nested code blocks would look like within Python.
A nested code block is a code block that’s contained within another code block.
To deal with nested blocks, you need to add another level of indentation for each additional nested code block.
if 8 > 2:
print("Eight is greater than two!")
if 8 < 10:
print("Eight is less than ten!")
if 8 < 9:
print("Eight is less than 9!")
Copy
Comments in Python
Commenting your code is a good habit to get into as it can help others and yourself understand what your code was intended to do.
No matter how well written your code is, sometimes it can be incredibly hard to work out what particular parts of your code was intended to do. Commenting helps alleviate that problem.
Single Line Comments
To add a single line comment to your code, you need to add the number sign “#
” to the front of the line.
#This is an example comment
print("Hello World!")
Copy
Multi Line Comments
As the Python interpreter ignores new lines within a triple-quoted string (''' String '''
) we can utilize it as a way of handling multi line comments efficiently.
'''
This is a sample
of multiple line comments
using triple quotes
in Python
'''
Copy
Using this, you can save the effort of adding the number sign (#
) to every line of text.
Variables In Python
In this section we will by giving you a quick run-through on variables.
If you want to learn more about variables you can check out our Variables in Python Guide.
In Python, you do not have to worry about assigning variables a specific type, such as an integer (Number) or string.
Defining a variable in Python is incredibly straightforward.
All you need to do is write the variable name, followed by the equals symbol =
and then finally the value you want to set to that variable.
exampleVariable = "Hello World"
Copy
Above is an example of a defining a variable called exampleVariable
containing a string in Python.
Multi-Line Statements
While statements are typically ended in Python by a new line, it is possible to split them into multiple lines.
To do this, you need to make use of the continuation character ( \
) to denote where the line should split and continue.
For example, if you wanted to split a simple addition line so that it is more readable, then you can do the following.
total = value_one + \
value_two + \
value_three
Copy
If you are dealing with arrays or dictionaries, then you don’t need to worry about using the continuation character.
Python automatically handles arrays ( [ ]
) and dictionaries ( { }
) spanning across multiple lines.
The Python interpreter ignores new lines until the array or dictionary has been closed.
fruit = ['Banana', 'Apricot', 'Orange',
'Strawberry', 'Watermelon', 'Kiwifruit',
'Mandarin', 'Grapes', 'Pineapple']
Copy
Hopefully, you now have a basic understanding of Python’s syntax and have an idea of how Python code needs to be written.
In our next Python guide, we will be walking you through how Python deals with variables.
If you have any issues understanding the Python syntax or have any feedback, feel free to post a comment below.
Great explanations on syntax use. I am a beginner at Python so this will come in handy