In this tutorial, I go through the basics of Python lists and how you can use them.
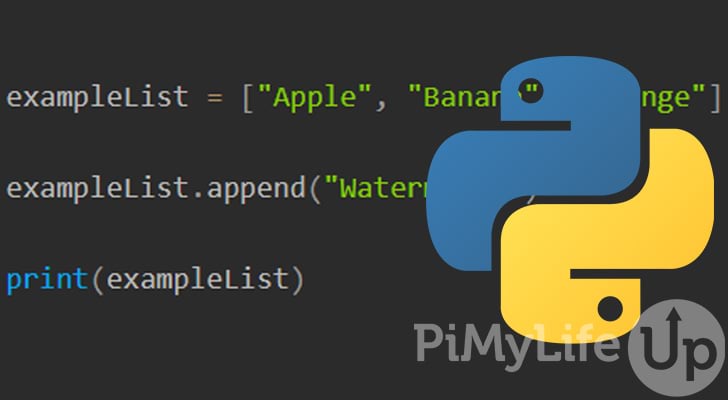
Lists are a data type in Python and store multiple items within a single variable. You can store multiple data types within a list, such as strings, integers, objects, or other data collection types. Lists also support duplicate data across multiple elements.
A list has a defined order that does not change. To explain further, being ordered means an element that exists will always be the same unless you direct Python to alter it.
Unlike Tuples, lists are mutable and can be altered after creation. We go through some of the many ways to alter the data within a list by using methods or operators.
It is important to note that Python does not have built-in support for regular arrays, using lists is recommended instead.
There are three other data types that you can use within Python. These include tuples, sets, and dictionaries. Each has its use case, but we focus on the list data type for this tutorial.
Table of Contents
- Creating a List
- Accessing Values within a List
- Adding Values into a List
- Updating Values within a List
- Copying a List
- Deleting Values within a List
- Deleting a List
- List Built-in Methods
- Conclusion
Creating a List
Creating a list within Python is straightforward and similar to creating an array in other programming languages.
To create a list in Python, simply place all your list elements inside square brackets, and separate the elements with commas. We will go through several examples below.
Creating a Blank List
To create an empty list, simply create a variable using empty square brackets. You can add some elements to this empty list using append, insert, or list.
exampleList = []
List of Integers
In this example, we create a list that contains purely just integers. A comma separates each element.
exampleList = [1, 2, 3]
List of Strings
You can make a list that contains multiple strings. Each string element must be contained within quotes and separated by a comma.
exampleList = ["Apple", "Banana", "Orange"]
List of Mixed Types
You can mix different data types within a list. In our example below, we have some strings and integers. We have a duplicate value in the list as well.
exampleList = ["Apple", 1, 2, "Banana", 1, "Orange"]
Multidimensional List
Our last example is a multidimensional list, sometimes referred to as a nested list.
In our example below, we have a total of three lists. The first list contains our two nested lists.
exampleList = [["Apple", "Banana"], ["Orange", "Watermelon"]]
Accessing Values within a List
There are multiple ways that you can access a value within a list. This section will touch on using an index, negative index, and slicing.
To access an element inside a list, we can use the index operator [index]
on our list variable. Much like an array, a list index starts counting from 0
. So, a list with 3
elements will start at 0
and finish at 2
.
You can iterate through a list programmatically by using the for loop. Alternatively, you can access an element directly by using the index number.
Using an Index
In our example below, we create a list with 4
elements containing strings. Since we count from 0
, the index finishes at 3
. Python will throw an error if you try to use an index outside this range.
We print two elements, the first string with an index of 0
and the third string with an index of 2
.
exampleList = ["Apple", "Banana", "Orange", "Pear"]
print(exampleList[0])
print(exampleList[2])
The output below displays both of our strings that are within the list.
python list.py
Apple
Orange
Multidimensional List
Accessing a multidimensional list is straightforward and not much different from accessing a single list.
In our example below, we have two nested lists within a list. To access the first list, we will need to use the index 0
. Then, next to that index, we specify the index we wish to access from the inner list.
So, if we wanted to access banana, we would write the following code exampleList[0][1]
.
In the example below we print an element from each inner list.
exampleList = [["Apple", "Banana"], ["Orange", "Pear"]]
print(exampleList[0][1])
print(exampleList[1][0])
Our output below shows that we accessed the second element in the first list and the first element in the second list.
python list.py
Banana
Orange
Using a Negative Index
If you want to work from the back of the list towards the front, you can use a negative index. When using a negative index, -1
refers to the last element in the list. So, the closer you go to the first element, the higher the negative number.
In our example below, we have a list of 4
elements with -1
being the last element and -4
being the first element. We access the last element (-1
) and the second element (-3
).
exampleList = ["Apple", "Banana", "Orange", "Pear"]
print(exampleList[-1])
print(exampleList[-3])
In the example below, we successfully accessed the correct elements.
python list.py
Pear
Banana
Slicing
If you want to access a range of elements rather than a single element, you can achieve this by using a technique known as slicing.
To slice, you will need to use the slicing operator, a colon (:
). On the left side of the colon, you specify the start, and on the right side, you specify the end.
The numbers you use for slicing are slightly different from using an index. For example, position 0
is before the first element, and position 1
is after it. So, if we wanted to select the first P
in our diagram below, our slice would be the following [0:1]
.
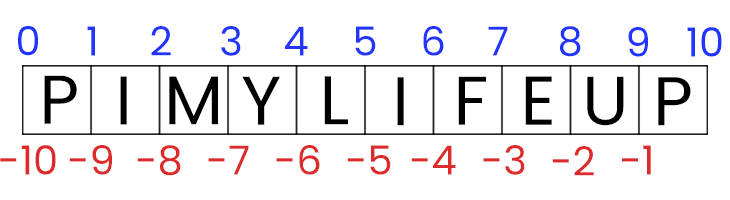
By not referencing a number in the slice, Python will assume from the start or end. For example. [:2]
will be everything from the start to position 2
. On the flip side, [3:]
will be everything from position 3 to the end.
In the code below, we do several examples of slicing. The first example is from positions 1
to 3
or [1:3]
. The second is from the start to positions 3
or [:3]
. Lastly, we have positions 1
to the end or [1:]
.
exampleList = ["Apple", "Banana", "Orange", "Pear"]
print(exampleList[1:3])
print(exampleList[:3])
print(exampleList[1:])
Below is the output from the three slices we performed in the above code. Remember, the slicing refers to positions between the elements and not the elements themselves.
python list.py
['Banana', 'Orange']
['Apple', 'Banana', 'Orange']
['Banana', 'Orange', 'Pear']
Adding Values into a List
You can add a value to a list using several different methods. We will touch on using the append, insert, and extend methods with Python lists.
Append Method
The easiest way to add a new value to an existing list is by using the append method. You can only add a single element at a time, and Python will always add it to the end of the list.
The syntax of the append method is below.
list.append(newValue)
In the example below, we append watermelon to our existing list.
exampleList = ["Apple", "Banana", "Orange"]
exampleList.append("Watermelon")
print(exampleList)
As you can see in the output below, watermelon is now part of our list.
python list.py
['Apple', 'Banana', 'Orange', 'Watermelon']
Insert Method
Using the insert method, you can add a new value anywhere within a Python list. When adding a new element into the list, all elements will be shifted right. So, for example, if you insert at index 3
, the existing value at index 3
will be shifted into index 4
and so on.
list.insert(position, newValue)
In our example below, we insert watermelon into our existing list at index 1
.
exampleList = ["Apple", "Banana", "Orange"]
exampleList.index(1, "Watermelon")
print(exampleList)
As you can see in the output below, watermelon is now at index 1
.
python list.py
['Apple', 'Watermelon', 'Banana', 'Orange']
Extend Method
Extend is similar to append but can add multiple elements. However, it can only add the elements at the end of the list. Below is the syntax for the extend method.
list.extend(elements)
We add three new elements to our existing list in our example below. Python will add these to the end of the list.
exampleList = ["Apple", "Banana", "Orange"]
exampleList.extend(["Watermelon", "Pear", "Apricot"])
print(exampleList)
The output below shows that our new elements have been added to our existing list.
python list.py
['Apple', 'Banana', 'Orange', 'Watermelon', 'Pear', 'Apricot']
Please Note: You can use the extend method with other data types such as tuples, sets, and dictionaries.
Updating Values within a List
It is possible to alter existing elements within a list. There are two methods that you can use, which I cover below.
Update a Single Value
You can easily update a single element by using the =
operator. In the example below, we update banana to apricot.
exampleList = ["Apple", "Banana", "Orange"]
exampleList[1] = "Apricot"
print(exampleList)
Running the script above should give you an output exactly like the example below.
python list.py
['Apple', 'Apricot', 'Orange']
Update a Range of Values
It is also possible to update a range of values using the same slicing method we mentioned in the accessing values section.
It’s important to remember that the numbers you use for slicing are slightly different from using an index. For example, position 0
is before the first element, and position 1
is after it.
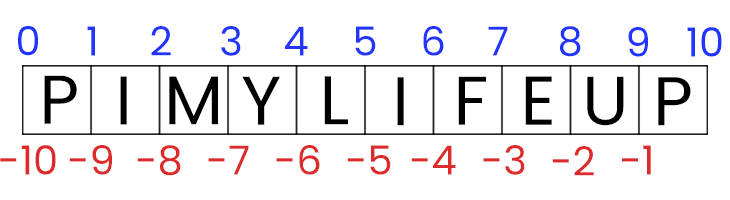
In the example below, we change the last 2 elements to new values.
exampleList = ["Apple", "Banana", "Orange"]
exampleList[1:3] = ["Apricot", "Pear"]
print(exampleList)
Our output below shows that the last two elements have been replaced with our new values.
python list.py
['Apple', 'Apricot', 'Pear']
Python will insert any extra elements next to the replaced elements if you specify more elements than required. The code will shift the existing elements to the right to make room for the new elements.
The example below has 4
new elements, but we have only specified 2
to be replaced.
exampleList = ["Apple", "Banana", "Orange"]
exampleList[0:2] = ["Apricot", "Pear", "Watermelon", "Grapes"]
print(exampleList)
In the output below, you can see that apricot and pear replaced apple and banana. However, next to them are our other two new elements and the remaining original element, orange.
python list.py
['Apricot', 'Pear', 'Watermelon', 'Grapes', 'Orange']
Copying a List
You may sometimes need to copy a list to a new variable. However, you cannot simply use the =
operator as that will only create a reference to the original list. Instead, to copy a list to a new variable, you will need to use the copy method or list constructor.
Copy Method
Using the copy method is pretty straightforward and is the method that I recommend using.
In the example below, we simply copy our existing list into a new variable named newList
.
exampleList = ["Apple", "Banana", "Orange"]
newList = exampleList.copy()
print(newList)
The print output displays all the elements that have been copied from exampleList
to newList
.
python list.py
['Apple', 'Banana', 'Orange']
List Constructor
You can use the list constructor to create a new list from an existing list. It is pretty straightforward, as you can see in the following example.
exampleList = ["Apple", "Banana", "Orange"]
newList = list(exampleList)
print(newList)
In our output below, you can see that Python copied our list correctly to a new list.
python list.py
['Apple', 'Banana', 'Orange']
Deleting Values within a List
There are several ways to delete values from an existing Python list. This section, will touch on using the remove, pop, del, and clear methods.
Remove Method
Using the remove method, you can remove a specific element from the list. If the element does not exist, Python will throw an error.
The remove method will only delete the first occurrence of the specified element, so you may need to run it multiple times if there are duplicate elements.
list.remove(element)
In the example below, we remove the first element, which contains apple, which just happens to be our first element.
exampleList = ["Apple", "Banana", "Orange"]
exampleList.remove("Apple")
print(exampleList)
The output below shows that the element containing apple has been removed, and there are now only two other elements.
python list.py
['Banana', 'Orange']
Pop Method
The pop method accepts a single optional parameter. The parameter is the index value for the element you wish to remove. Python will remove the last element if you do not enter a parameter (index).
list.pop(index)
For this example, we will remove orange, which has an index value of 2
.
exampleList = ["Apple", "Banana", "Orange", "Watermelon"]
exampleList.pop(2)
print(exampleList)
As you can see in our output below, orange has been removed from the list.
python list.py
['Apple', 'Banana', 'Watermelon']
Clear Method
The clear method is perfect if you want to wipe the entire list but do not want to remove the list variable. Clear does not accept any parameters.
list.clear()
In the example below, we create a list and then run the clear method.
exampleList = ["Apple", "Banana", "Orange"]
exampleList.clear()
print(exampleList)
When we run our Python file, we see output indicating that the list has no elements, and just two square brackets with nothing inside.
python list.py
[]
Del Keyword
The del keyword can be used to remove specific elements from the list. This method is similar to pop but requires you to specify the element you want to delete. If you do not specify an index, del will delete the entire list.
exampleList = ["Apple", "Banana", "Orange"]
del exampleList[0]
print(exampleList)
Since we specified index 0
, the element containing apple is deleted.
python list.py
['Banana', 'Orange']
Deleting a List
Using the del keyword, you can delete a list entirely. After the list has been deleted, any call to the list will result in an error unless you recreate it.
The example below demonstrates how to delete a list.
exampleList = ["Apple", "Banana", "Orange"]
print(exampleList)
del exampleList
print(exampleList)
The first print will work perfectly fine if you run the above script. However, the second print will throw an error as we deleted the list.
python list.py
['Apple', 'Banana', 'Orange']
Traceback (most recent call last):
File "D:\Python\list.py", line 7, in <module>
print(exampleList)
NameError: name 'exampleList' is not defined
Python List Built-in Methods
There are quite a few different methods that you can use on a Python list. We have touched on most of the methods in this Python list tutorial, but there are more that you might need to use.
I will quickly list a few that you are likely to use.
list.append(element) | Will add a new element at the end of the list. |
list.clear() | Clears all the elements from the list, leaving you with an empty list. |
list.copy() | Returns a copy of the list. |
list.extend(list2) | Adds multiple elements to the end of the list. You can add lists, tuples, sets, or dictionaries. |
list.index(element) | Returns the index of the first element that matches the specified value. |
list.insert(index, element) | Inserts an element into the list at the specified index. |
list.pop(index) | Removes the element at the specified index. Otherwise, it removes the last element. |
list.remove(element) | Removes the first element that matches the specified value. |
list.reverse() | Reverses the order of the elements in the list. |
list.sort() | Sorts the list. |
Conclusion
I hope that you now have a good understanding of using lists in Python. You will likely use lists quite a bit when writing code in Python, as they are incredibly powerful. Also, don’t forget about the other data types, such as tuples, sets, and dictionaries.
There is a ton more to learn about Python, so I highly recommend that you check out some of our other Python tutorials. They are a great way to learn the basics of Python quickly.
Please let us know if there are any improvements we can make to this tutorial by leaving a comment at the bottom of this page.