In this tutorial, we will take you through how to use the join() method to combine multiple strings in the Python programming language.
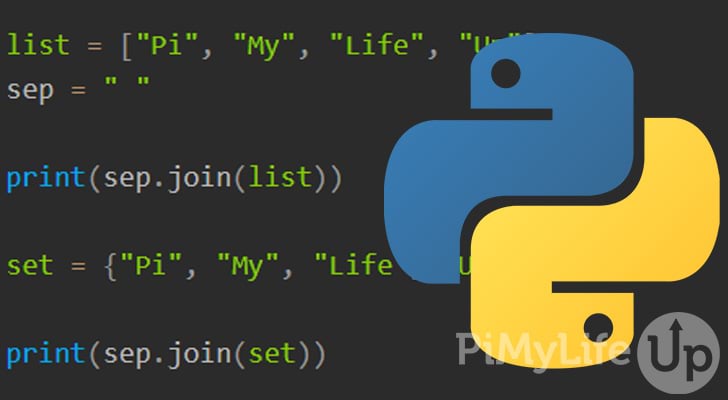
The join method makes it easy to combine multiple strings stored within an iterable such as tuples, lists, sets, and dictionaries. In addition, you can set characters to be used as a separator, such as a space or a dash.
There are many more methods that you can use to manipulate strings within Python. For example, you can use the split method to split a string based on a specified separator.
The tutorial below will touch on the syntax of the join method and the various iterables you can use with join. For example, we will touch on using a join with a list, dictionary, set, or tuple.
Syntax of the Python join() Method
The syntax of the join method is straightforward as it only has a single parameter. We will briefly explain each part of the method below.
string.join(iterable)
Copy
- The string is the separator to use between each string. You will likely use a space, but any combination of characters will work.
- You will need to use an iterable as the argument. For example, a dictionary, list, tuple, or set will work. However, ensure that the iterable contains string values; otherwise, a TypeError exception may be thrown.
The return value will be a string containing all the values of the iterable, with each value separated by our defined separator.
Examples of Using the join() Method in Python
To demonstrate how the join() method works, we will go through a few different examples using a range of different data types.
You can run each of the examples on your local machine, as long as you have Python installed. You can install Python on Windows, Linux, macOS, and Raspberry Pi.
Using join() on a list
Our first example will use the list data type as the iterable that contains our different strings. We will also use a space as the string separator.
list = ["Pi", "My", "Life", "Up"]
sep = " "
print(sep.join(list))
Copy
The print function outputs the following string to the terminal. Our final string is all strings from the list separated by a space.
python join.py
Pi My Life Up
Copy
Using join() on a dictionary
In this second example, we will use the dictionary data type. Dictionaries use key-value pairs, but join will only use the key values. So, if a key contains non-string values, a data TypeError will likely occur.
In our Python code below, we combine all our dictionary keys but will be separated by “->
“.
dict = {
"fruit": "Apple",
"healthy": True,
"calories": 95,
"colors": ["red", "yellow", "green"]
}
sep = "->"
print(sep.join(dict))
Copy
The following concatenated string will output to the terminal thanks to the print function. As you can see, each of our strings is separated by ->
.
python join.py
fruit->healthy->calories->colors
Copy
Using join() on a set
In this example, we will use a set as the iterable in the join method. Since a set is unordered, the output will likely be random on every run-through of the script.
set = {"Pi", "My", "Life", "Up"}
sep = " "
print(sep.join(set))
Copy
As you can see in our output below, the join method combined our strings contained within the set. As I mentioned earlier, the output will be random as the data is stored in a set.
python join.py
Life My Pi Up
Copy
Using join() on a tuple
It is important to note that a tuple data type is very similar to a list. The main difference between a list and a tuple is that a tuple is immutable after creation.
tup = ("Pi", "My", "Life", "Up")
sep = " "
print(sep.join(tup))
Copy
Since a tuple is ordered, our output is in the correct order and separated correctly.
python join.py
Pi My Life Up
Copy
Conclusion
I hope this tutorial has given you a decent idea of how you can use the join method to combine multiple strings into a single string. You will find it extremely useful if you handle many strings in your Python script.
If you are new to Python and want to learn more, I recommend checking out the tutorial on Python strings. It will take you through many of the fundamentals of using strings in Python. You might also be interested in learning how to convert an int into a string.
If we can improve this tutorial, please do not hesitate to let us know by leaving a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support