In this tutorial, we will be showing how you can convert an int to a string in the Python language.
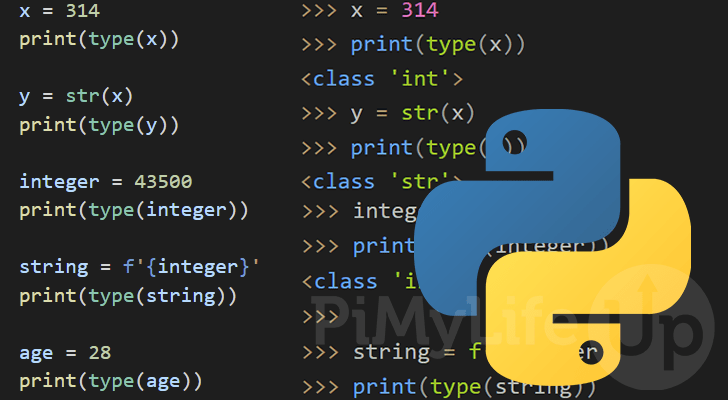
As we talked about in our variables in Python guide, Python dynamically assigns a type to a variable when created.
However, it is possible to change between data types using various built functions. For example, this is useful when you have ended up with a number type such as an integer but need the value to be a string.
For this guide, we will be showing how you can convert an integer to a string using Python’s in-built functionalities.
Over the following sections, we will explore four different methods you can utilize to convert your number to a string.
For most cases, you will want to use the “str()
” function. However, it is always helpful to know other ways of dealing with simple tasks.
Table of Contents
- Convert an Integer to a String using Pythons str() Function
- Using f-strings to Convert an Integer to String
- Convert an Integer to String using Pythons % Operator
- Using the .format() function to convert an Integer to a String
- Conclusion
Convert an Integer to a String using Pythons str() Function
For our first method, we will be exploring the most obvious way to convert an integer to a string within Python. That is to utilize the str()
function.
The str()
function allows you to convert a Python object into its string representation. It is straightforward to use as all you need to do is pass your integer into it.
For example, the most basic syntax for this function would be to use it, as we have shown below, where “INTEGER
” is your number, or a variable containing a number.
str(INTEGER)
Copy
Example of using str() in Python to Convert an Int to a String
Now that we know there is a function we can use in Python to convert an int to a string let us put it to use.
For this example, we will create a simple variable called “x
” and assign it the value 314
.
We will first print the type of this function to show that it is, in fact, an integer. Next, we use the str()
function to convert the int and store that new string in the y
variable.
We can use Python’s print()
, and type()
functions to tell us the variables type. type()
is used to retrieve the variable’s type, and print()
is used to print the type to the terminal.
x = 314
print(type(x))
y = str(x)
print(type(y))
Copy
Below you can see after running these lines in the Python interpreter, our initial number “x
” was converted to a string and stored in “y
“.
>>> x = 314
>>> print(type(x))
<class 'int'>
>>> y = str(x)
>>> print(type(y))
<class 'str'>
Copy
Using f-strings to Convert an Integer to String in Python
Python 3.6 introduced a new feature to the language called “f-strings”. An f-string allows you to insert variables directly within the string itself.
For this method, you must use the f
character before starting the string. The string must also use single quotes (' '
), not double-quotes. Then within that string, you can reference your variable by encasing it in curly braces ({}
).
When the Python interpreter reads this string, it will automatically expand all the values into strings.
Below you can see how simple this method is to utilize. It is even slightly faster than using the str()
function.
f'{INTEGER}'
Copy
Example of using f-string to Convert an Int to string
For this example, we will start by creating a variable called “integer” and storing the number “43500” in it.
Next, we create a new f-string and reference our integer variable within it, encasing it in curly brackets. Finally, the resulting formatted string is stored within a variable called “string
“.
With this example, you will see how easily Python can convert an int to a string. It is also much cleaner to use than the methods referenced in the later sections.
To show you the type conversion we use the print()
and type()
functions on each variable.
integer = 43500
print(type(integer))
string = f'{integer}'
print(type(string))
Copy
Below is the sort of output you should get after running the above example. You can see how Python’s f-string syntax easily converted our integer to a string.
>>> integer = 43500
>>> print(type(integer))
<class 'int'>
>>>
>>> string = f'{integer}'
>>> print(type(string))
<class 'str'>
Copy
Convert an Integer to String using Pythons % Operator
Using the str()
function is not the only way to convert an integer to a string within Python.
One of these other methods us using Python’s percentage sign operator (%s
) to substitute your number into the string. This format works best when you want to insert an integer into a string.
When writing a string, use “%s
” in place of where Python should insert the number. You can then reference this variable outside the string using the percentage sign, followed by the variable’s name.
For example, the most basic way of using this method is shown below. The “%s
“, will be replaced by the value stored in the “INTEGER
” variable.
"%s" % INTEGER
Copy
Example of using Pythons % Operator to Convert an Int to String
For this example, we will be writing a simple string where we want the integer stored in our “age
” variable converted to a string.
We start this Python script by assigning the number 28
to our “age
” variable. We will use both print()
and type()
to confirm the data type.
After this, we use a simple string with only “%s
” in it. The percentage sign operator is used after, followed by our variable.
The Python interpreter will process this, see that we expect string (Thanks to %s
), and convert our age variable before storing it in the variable called “string
“.
age = 28
print(type(age))
string = "%s" % age
print(type(string))
Copy
If you run this code within the Python interpreter, you will see how it has converted our int to a string.
>>> age = 28
>>> print(type(age))
<class 'int'>
>>>
>>> string = "%s" % age
>>> print(type(string))
<class 'str'>
Copy
Using the .format() function to convert an Integer to a String
We can also use the .format()
function to convert our integer to a string within Python. The behavior of this is similar to using the %
operator.
Python’s “.format()
” function allows us to insert variables into a string where placeholders are present.
Using a string with only the “{}
” placeholder, you can easily convert your string to an int. With this, we only need to pass our int into the format()
function.
"{}".format(INTEGER)
Copy
Example of using .format() to Convert an Int to a String
For this example, let us assign the integer “214
” to a variable called “number
” and convert it to a string.
Using the “.format()
” method for converting an int to a Python string is very straightforward.
All you need is a string with a placeholder ({}
), and then the format function with your number passed in. During the formatting process, Python will convert out integer to a string.
number = 214
print(type(number))
string = "{}".format(number)
print(type(string))
Copy
Using these within Python you can see the type change from an integer, to a string once its passed into the “.format()
” function.
>>> number = 214
>>> print(type(number))
<class 'int'>
>>>
>>> string = "{}".format(number)
>>> print(type(string))
<class 'str'>
Copy
Conclusion
Throughout this guide, we have shown you a few different ways to convert an integer to a string within Python.
For most use cases, the str()
function will be the best choice. It is easy to use and clear on what it’s doing.
The other methods involve inserting your number into a string, during which it is converted into a string itself.
If you have had any issues with these methods, please comment below.
Be sure to also check out our many other Python tutorials to learn the language better. We also have a wealth of tutorials on other programming languages.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support