Global Variables in Python are a relatively straightforward but important concept to learn when first starting with Python.
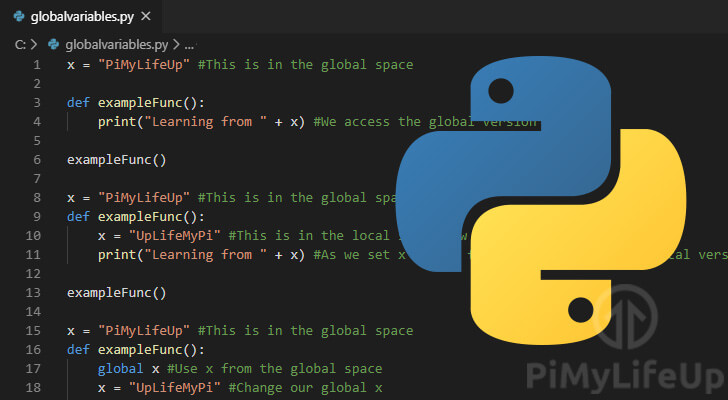
In this Python tutorial, we will be exploring what global variables are in Python and how you should deal with them.
If you are starting with Python, then we recommend that you start with our getting started with Python guide.
Before you start this guide, you should first have a basic understanding of what a variable is in Python.
If you need a quick refresher on what a variable is in Python, then be sure to follow our Variables in Python guide.
Before we get started, you should know that in Python, there are both local and global variables.
Local variables are variables that are defined within a function and are only available to that function while it runs.
When the function finishes, the local variable will be removed from memory and cease to exist.
Global variables are variables that have been defined outside of any function. These variables will exist until they are deleted or the program ends.
x = "pimylifeup" #This is a Global variable
def exampleFunction():
b = "uplifemypi" #This is a Local variable
Copy
The example above should give you a quick understanding of when a variable can be considered global or local.
Python Global Variables
In Python, all variables that are created outside of a function are considered to be in the “global” space.
Anything within the script can access these global variables, and this includes code inside functions in your script.
Below we have an example of accessing a global variable from within a function and printing out its value.
x = "PiMyLifeUp" #This is in the global space
def exampleFunc():
print("Learning from " + x) #We access the global version of x
exampleFunc()
Copy
You can see with this example that we access our global variable x
from within the function and output its value.
Learning from PiMyLifeUp
However, there is a caveat to using global variables within functions.
As soon as you try to assign a value to a global variable without using the global keyword, that value becomes a local variable, and the global variable is ignored.
By becoming a local variable, only the function itself can access its value. The original global variables value is therefor, not modified.
We can observe this behavior by writing a small script where we try to change the value of x
within the function.
x = "PiMyLifeUp" #This is in the global space
def exampleFunc():
x = "UpLifeMyPi" #This is in the local space now
print("Learning from " + x) #As we set x locally, we access its local version
exampleFunc()
print("Learning from " + x)
Copy
Upon running this code, you will notice that both print()
functions outputted different values as expected.
Learning from UpLifeMyPi
Learning from PiMyLifeUp
It is possible, however, to still modify a global variable within a function by making use of the global
keyword.
Using the Global Keyword in Python
To be able to modify global variables within a function, you must first make use of the global
keyword.
This keyword tells Python that the variable should be accessed from the global space and not the local one.
For example, if we take the script we used in the previous section and add global x
to the start of the function, you can see that the value of x
is now modified in the global space.
x = "PiMyLifeUp" #This is in the global space
def exampleFunc():
global x #Use x from the global space
x = "UpLifeMyPi" #Change our global x
print("Learning from " + x) #Print out the global x value
exampleFunc()
print("Learning from " + x)#Print out the global x value again
Copy
As you can see from the result of this function, both print()
functions are now returning the same value.
This result is because we made use of the global
keyword, and are now modifying the global variables value from within the function rather than creating a new local variable.
Learning from UpLifeMyPi
Learning from UpLifeMyPi
Using the global keyword within a function, you are also able to define new global variables.
While this isn’t typically recommended, it is possible to do quite easily.
All you need to do is use the global
keyword, as we did in the code example before.
The variable is automatically created on the global scope as soon as a value is assigned to it.
def exampleFunc():
global x #Use x from the global space
x = "PiMyLifeUp" #Set the value of the global x variable
exampleFunc()
print("Learning from " + x)#Print out the value of the global x value
Copy
With this example code, you can see that we first create our x
variable within our exampleFunc()
function then access this same variable using print()
in the global space.
If you were to do this without using the global
keyword, you will run into an error as the variable would never exist outside of our exampleFunc()
function.
However, thanks to our use of global
, we can access the variable easily as the x
variable is made available in the global space.
You should get the following result from the example code above.
Learning from PiMyLifeUp
At this point, you should now have an understanding of the difference between a local and a global variable in Python.
You should also now know how to make use of global variables within a function and know how to use the global
keyword to be able to modify them.
If you have any questions or any feedback on this Python global variables tutorial, then feel free to drop a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support