In this tutorial, we will take you through each of the logical operators that you can use in Python.
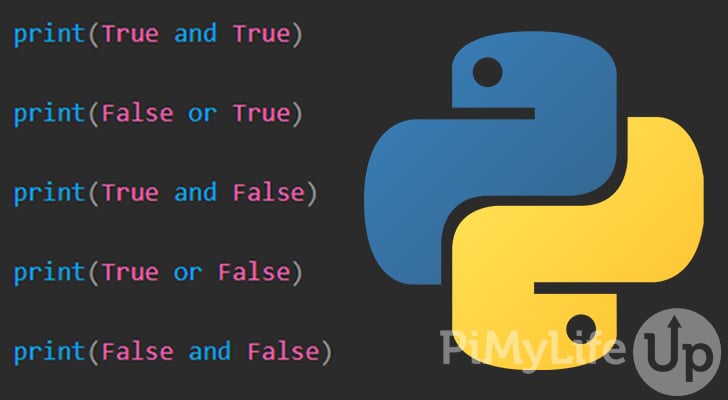
In Python, a logical operator performs operations on the output of two conditional statements (Operands). The output will be either true or false.
Using logical operators allows you to perform a check on two operands. For example, you can check if both operands are true or if only one of them is true. You can create some smart logic for controlling your Python script using these operators.
It makes perfect sense to get a good understanding of all the different types of operators in Python. However, for this tutorial, we will be purely focusing on logical operators.
The table below showcases each of the logical operators you can use. It is an excellent reference if you need to remind yourself of the functionality of each operator.
Name | Example | Result |
---|---|---|
AND | x and y | true if both x and y are true |
OR | x or y | true if x or y is true |
NOT | not x | true if x is false |
The above table is a very simplistic explanation of each logical operator you can use in Python. We go into more detail on each operator further down this page.
This tutorial covers the (logical) Boolean operators, which are different from the bitwise operators. We also assume you are using Python 3 and not any older versions of Python where syntax and terminology may differ slightly.
Example of Logical Operators in Python
In this section, we will take you through each of the logical operators in more detail. We will show you examples of how you can use these operators in your next Python program.
We will often refer to operands in this tutorial, which are the objects, quantity, or Boolean values being evaluated by our operation. For example, the x
and y
in the following expression are the operands, and the “and
” is the operator.
x and y
Copy
Logical and
Operator in Python
The “and
” operator in Python allows you to compare two operands. If both operands are True
, the operator will return True
. If either value is False
, the operator will return False
. Lastly, if both operands are False
, the operator will return False
.
The syntax is very straightforward, with the operator “and
” placed between two different operands.
x and y
Copy
By using the “and
” operator, the result will only be true
when x
and y
both are true
.
The table below shows the different outcomes of using the “and
” operator with different values for x
and y
.
x | y | x and y |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
When evaluating the “and
” operator, Python will check the left operand before it checks the right operand. There is also precedence between the different types of logical operators.
Code Example of the and
Operator
This section will demonstrate how the “and
” operator works in Python. We use the print function to output the result of each of our operations.
- Our first print function will return
True
as both operands areTrue
. - The second print will return
False
as only one operand isTrue
and the other isFalse
. The “and
” operator requires both operands to beTrue
. - Lastly, the third print will return false as both operands are
False
.
print(True and True)
print(True and False)
print(False and False)
Copy
The output below shows the results of each of our “and
” operator examples.
True
False
False
Logical or
Operator in Python
You can use the “or
” operator in Python to compare two operands to see if either is True
. The operator will return True
if at least one operand is True
.
The syntax is very straightforward, with the operator “or
” placed between two different operands.
x or y
Copy
The table below demonstrates the different outcomes of using the “or
” operator to compare two operands. The only time that or returns False
is when both x
and y
are False
. Every other combination will result in a True
result from the “or
” operator.
x | y | x or y |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
When evaluating the “or
” operator, Python will check the left operand before it checks the right operand. There is also precedence between the different types of logical operators.
Code Example of the or
Operator
In the example below, we have some Python code demonstrating how the “or
” operator functions. We will go through each of the examples and the result they will return.
- The first print will return
True
as both of our operands areTrue
. - Our second print will return
True
as one of our operands isTrue
. The “or
” operator allows one of the operands to beFalse
. - Lastly, our third print will return
False
as both of our operands areFalse
.
print(True or True)
print(True or False)
print(False or False)
Copy
If you run the above Python script, you should get an output similar to the one below.
True
True
False
Logical not
Operator in Python
The “not
” operator allows you to flip the result of an operand. So, if an operand returns True
, the “not
” operator will change the result to False
.
You can only use the “not
” operator on a single operand, so you will need to use it multiple times if you need to apply it on multiple operands.
The syntax of the “not
” operator is straightforward within Python as it is written simply as “not
“. Below is an example of how you can use the operator.
not x
Copy
The table below demonstrates how the “not
” operator will handle an operand that is true or false.
x | not x |
---|---|
false | true |
true | false |
Code Example of the not
Operator
In the example below, we have two examples of how the not operator will function with an operand.
- The first print will simply return the inverse of
x
, which will beFalse
. - Our second example contains the “
and
” operator that normally returnFalse
becausey
is false. However, by using the “not
” operator ony
, we get the inverse, soy
isTrue
; thus, our “and
” operator will also returnTrue
.
x = True
print(not x)
x = True
y = False
print(x and not y)
Copy
Running the above code will result in the following output into the terminal.
False
True
Logical Operator Precedence
Python will evaluate code from left to right and applies the same for grouping operators. However, logical operators have a precedence ordering, so one operator may take precedence over another operator. It is important to understand the precedence as it may impact the outcome of your conditional statements.
Our logical operators are ranked in the following order.
- not
- and
- or
In our example below, we show a few examples of how the logical operator precedence might impact the outcome of your code.
- Our first print statement contains a not operator. The “
not
” operator is evaluated first, so our output will beTrue
. The expression is evaluated as(True and (not False))
- The second print statement contains both an “
and
” operator and “or
” operator. Since “and
” is higher up in precedence, it will be evaluated first, resulting in an output ofTrue
. The expression is evaluated as((False and False) or True)
- Lastly, our third print is similar to our second print, but the expression is flipped. Since the “
and
” is evaluated first, the “or
” operator will guarantee our return value isTrue
. The expression is evaluated as(True or (False and True))
.
print(True and not False)
print(False and False or True)
print(True or False and False)
Copy
Running the Python code above will result in the output below.
True
True
True
Conclusion
I hope by now that you have a decent understanding of how Python logical operators will work with different operands. We discussed the “and
“, “or
“, and “not
” operators alongside a few different examples. Also, we discussed the precedence of the operators and how Python will execute them.
We have plenty more Python tutorials that I highly recommend checking out if you want to learn more about Python. For example, you might find our tutorial on if else statements useful if you are new to coding in the Python programming language.
Please let us know if you notice a mistake or an important topic is missing from this guide.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support