In this guide, we will be showing you how you can use the comma operator in JavaScript.
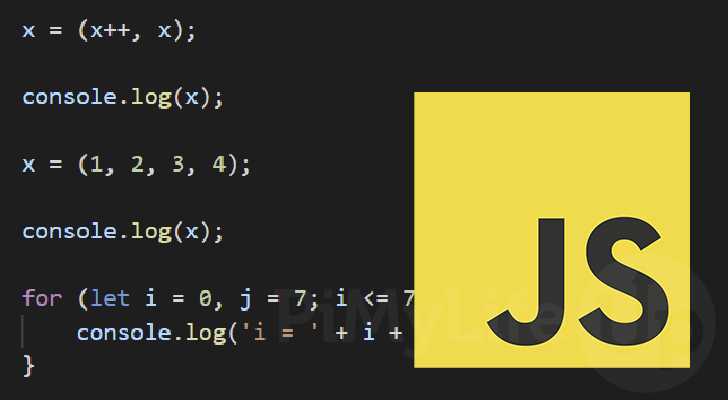
In JavaScript, the comma operator (,
) allows you to create a compound expression where you have multiple expressions being evaluated. The comma is used to separate each expression.
JavaScript evaluates the comma operator from left to right, with the right most expression being the value that is passed along.
While there are various use cases for this operator, it is especially useful for providing extra parameters to the for loop. For example, you can update multiple values every loop, as we will show later.
Over the following few sections, we will show you how to utilize this operator within JavaScript.
Syntax of the Comma Operator in JavaScript
For this first section, we will show you the comma operator’s syntax.
The comma operator is reasonably straightforward to use. All you need to do is use the comma symbol (,
) between each expression. You can chain as many expressions as you want together.
Below is an example of how you would use the comma operator to compound several expressions. An expression can be a value, variable, operator, or function call.
expr1, expr2, expr3, expr4...
Copy
Remember that the value returned from the last expression is what will be returned from the compound.
One thing to note is that you shouldn’t get confused between the comma operator and commas used within function arguments, parameters, and arrays.
Using JavaScript’s Comma Operator
Now that we have explored the comma operator’s syntax let us showcase how it is used within some actual code.
The following sections will explore a couple of ways to use the comma operator within JavaScript.
Basic Usage of the Comma Operator in JavaScript
Let us start by exploring a basic usage of the comma operator within JavaScript. This example will focus on showing you the operator’s left-to-right evaluation behavior.
We will explain this in two parts to give a better understanding of what is happening with this example. Let us start with the top half of this script.
We start by declaring a variable called “x
” and assigning it the value of 10
to give us a starting value.
On the following line, we have our first usage of the comma operator to assign a value to our “x
” variable.
- We use the post-increment operator (
x++
) for the first expression. - For the second, we simply reference our “
x
” variable.
Using the post-increment operator by itself would mean our “x
” variable would return its original version before being updated.
Since we use the comma operator, JavaScript will return the incremented value stored in our “x
” instead. This is because it is referenced last in our compound expression.
We will log the result of this compound expression to the console.
The second half of this code shows you how the left-to-right evaluation works.
Our final comma operator usage is super straightforward. Here we are simply using the comma operator to separate four different values.
Since JavaScript evaluates the comma operator from left to right, the final expression is what will be assigned to the variable.
The final value is output to the console by passing it into the console.log() function.
let x = 10;
x = (x++, x);
console.log(x);
x = (1, 2, 3, 4);
console.log(x);
Copy
After running the above example, you should see the following within your console. Here you can see how the comma operator affected the value stored within the “x” variable.
Simply put, the far-most right expression is what was returned by the expressions.
11
4
Copy
Using the Comma Operator within a for Loop
As mentioned earlier in this guide, the for loop is one of the most useful places to use the comma operator in JavaScript.
The reason for this is that you can update multiple values at once within the loops structure. As a result, you will notice that the comma operator will behave slightly differently from our previous examples.
Our first usage of the comma operator is within the initial expression of the for loop. Here we use the comma operator to declare the “i
” variable with the value of 0
and the “j
” variable with the value of 7
.
The second time we use the comma operator is in the increment expression. Again, we use the comma operator to increment the value of the “i
” variable and decrement the value of the “j
” variable.
Every loop we log the value of the “i
” and “j
” variables so you can see how the values are increased and decreased upon every loop.
for (let i = 0, j = 7; i <= 7; i++, j--) {
console.log('i = ' + i + ', j= ' + j);
}
Copy
After running the above example you will end up with the following result. From this result you can see how we were able to update
i = 0, j= 7
i = 1, j= 6
i = 2, j= 5
i = 3, j= 4
i = 4, j= 3
i = 5, j= 2
i = 6, j= 1
i = 7, j= 0
Copy
Conclusion
Throughout this tutorial, we have shown you how to use the comma operator in JavaScript.
This is a useful operator that allows you to compound multiple expressions within one expression.
Please comment below if you have any questions about using this operator.
Check out our many other JavaScript tutorials if you want to learn more about the language. We also have numerous other programming tutorials for those wanting to learn a different language.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support