This tutorial will show you how to use the console.log() function within JavaScript.
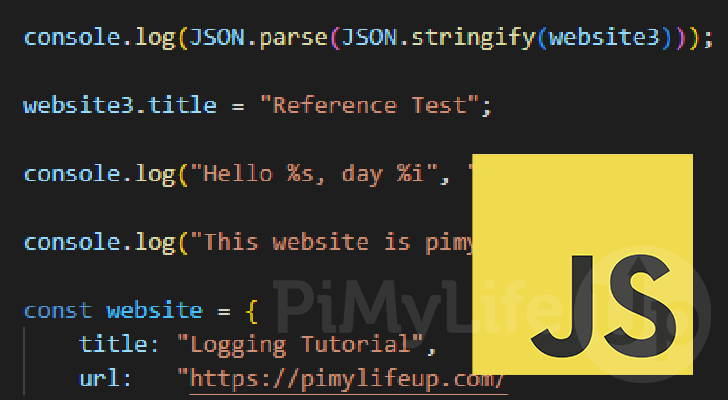
The console.log() function is an incredibly useful part of JavaScript that you can use to debug your code.
This function allows you to write messages to the console from your JavaScript. It allows you to easily debug your code on the fly as you can print out any required values when a problem occurs.
Best of all, almost all desktop web browsers have support for the console, and you can easily open it by pressing F12 on your keyboard.
Over the following sections, we will show you how exactly you can use this function and show how useful it can be.
You will quickly find that using console.log() in JavaScript is straightforward and critical to debugging code.
Syntax of the console.log() Function in JavaScript
In this section, we will be quickly exploring the syntax of the console.log() function in JavaScript. The syntax tells you a couple of things, such as what parameters the function requires.
The first thing we should mention is that the log() function is a method of the “console
” object. This object is implemented by web browsers or other clients and allows us to print text to the console.
Below you can see JavaScript’s syntax for the console.log() function.
console.log(message/object ...,);
This function can take in multiple parameters, but you can use as little as one.
JavaScript will print the values you pass into this function to the console. This value can either be a string containing a message or an object.
- Objects: If you pass in an object to the
console.log()
function, its values will be printed to the console.
However, please note that modern web browsers might show a reference to the object, so the values may not represent what was set when you fired the log. There is a way to work around this that we will cover later.
You can pass in multiple objects in the one function call by separating them using a comma (,
). - Strings: As mentioned before, you can pass in a string instead of an object. JavaScript will print this string to the console.
If this string includes substitution strings, the following parameter will be used as part of the substitution.
No value is returned by the console.log() function.
Examples of using the console.log() Function in JavaScript
This section will show a few examples of how you can use the console.log() function within JavaScript.
Hopefully, by the end of this section, you will understand how best to utilize it and how the console.log() functions.
Logging a String
One key way you will use JavaScript’s console.log() function is to print messages to the console.
You can simply pass a string into the console.log() function. Using the function like this is useful when you need a message printed if a certain code path is reached or not reached.
For example, we have often used this to log which path our code ended up taking, or when something is causing the script to not execute completely.
To showcase this, let us write a short example where we use “console.log()
” and pass in the string “This website is pimylifeup.com
“.
console.log("This website is pimylifeup.com");
If you were to run this piece of code, you would end up with the following message printed to the console.
This website is pimylifeup.com
Logging the Contents of an Object
The console.log() function also displays the information stored within a JavaScript object. The entire object structure is logged to the console when logging an object.
Logging an Object as a Reference
To showcase logging objects, we will start our example by declaring an object using the const keyword with the name “website
. After declaring the variable we will pass the object into JavaScript’s “console.log()
” function.
When used this way modern browsers will log a reference to the object rather than a copy of the object at that point in time.
const website = {
title: "Logging Tutorial",
url: "https://pimylifeup.com/"
}
console.log(website);
After running this piece of JavaScript, you will have a result similar to what is shown below. You can see both the object’s properties and the prototype for this object.
title: "Logging Tutorial"
url: "https://pimylifeup.com/"
[[Prototype]]: Object
Objects are logged as References
Now while passing in an object to console.log() is a straightforward process, there is a catch to this. Modern web browsers typically only pass in a reference to the object.
This means that if the object is modified after you log it, the web browser will update the logged value.
To showcase this behavior, let us take the same example as before. But this time, after logging the object, we will change the “title
” property to “Reference Test
“.
Since we want to modify the object’s contents, we will declare it using JavaScript’s let keyword.
let website = {
title: "Logging Tutorial",
url: "https://pimylifeup.com/"
}
console.log(website);
website.title = "Reference Test";
Below you can see that even though we logged the object before changing the value, the console was updated with the new values.
title: "Reference Test"
url: "https://pimylifeup.com/"
[[Prototype]]: Object
Safely Logging Objects in JavaScript
It is possible to work around this by utilizing the JSON object. What we will do is convert the object to a string using “JSON.stringify()
” to convert the object to a string, followed immediately by “JSON.parse()
” to convert that string back into an object.
By doing this we are essentially creating a new copy of the object meaning changes made after the fact won’t affect the value you logged.
We put this to use in the following JavaScript example of using the console.log() function.
let website = {
title: "Logging Tutorial",
url: "https://pimylifeup.com/"
}
console.log(JSON.parse(JSON.stringify(website)));
website.title = "Reference Test";
After running the example above you should end up with the following text logged to your console. With this result, you can see how any changes made to the object didn’t ruin the original log.
title: "Logging Tutorial"
url: "https://pimylifeup.com/"
[[Prototype]]: Object
Logging with String Substitution
JavaScript also allows you to build longer, more complicated messages for console.log() by using string substitution.
When using string substitution the first parameter becomes your message. Every parameter after the first is the value you want to be subbed into your string.
Please note for every substitution string you have, you should have a value to be inserted into it. Additionally, the order is very important.
String Substitution Strings
Below are the strings that will be substituted by the “console.log()
” function when processing your message in JavaScript.
%o
or%O
– Substitutes in a JavaScript object. Within your console, you should be able to click this result to get more information. This behavior will differ depending on your web browser.%d
or%i
– This allows you to substitute in an integer. JavaScript will convert different number types during substitution.%s
– JavaScript will substitute these characters with a string.%f
– A floating-point number will be output in the place of this substitution string.
Please note if you are using Firefox you can also format the precision of numbers. You do this by using a period (.
) followed by the number of decimal places (floating number) or the number of digits (integers).
For example, with an integer, you could use “%.2d
” if you wanted a two-digit number in all cases.
Example of using String Substitution with console.log()
Now that you know the substitution strings, let us show you how to put them to use. With this, we will pass in a simple string with two substitutions.
The first substitution is a string (%s
), and the second is an integer (%i
). Remember the order you specify your substitutions as the following parameters will be swapped in using that order.
For our example, we specify the string “World
” as the second parameter and the number 20
as the third parameter.
console.log("Hello %s, day %i", "World", 20);
After running the above example, you should end up with the following logged to your console. You can see how JavaScript’s console.log() function swapped out the substitution strings with the values we specified in the function parameters.
Hello World, day 20
Conclusion
By this point in the guide, you should now hopefully have a good understanding of how you can use the console.log() function in JavaScript.
This function allows you to easily log messages to the console, which is especially useful for debugging your code.
Please comment below if you have questions about using the console.log() function.
Check out our many other JavaScript tutorials to learn more about this useful programming language. Also, if you want to learn a new programming language, we have many other coding guides.
Love all the great work that is put into these tutorial’s