In this tutorial, we will teach you how to write and use a while loop in JavaScript.
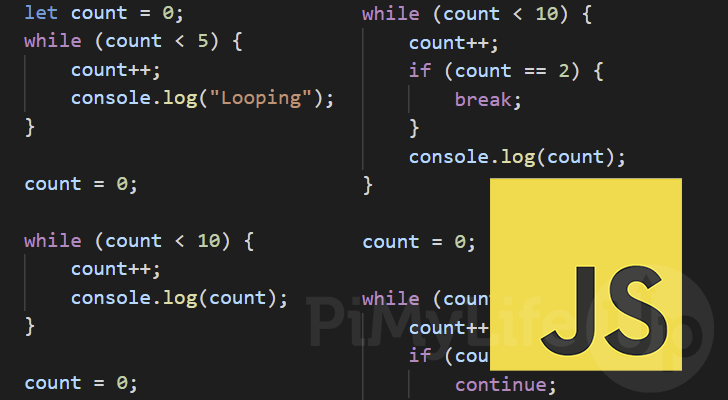
The while loop is one of the most straightforward loops supported by the JavaScript language. It allows you to continually execute a code block as long as a condition remains true.
A while loop is helpful when you need to continue to process data until a specific condition is true. You can also easily run a while loop indefinitely using an always true condition.
Using the while loop instead of the for loop in JavaScript is helpful when you don’t need to increment a value every loop.
Over the following few sections, we will show you how a while loop works and how to write it in JavaScript.
Table of Contents
Syntax of a while Loop in JavaScript
The while loop is straightforward to write and one of the easiest to remember as it only has a single condition to worry about.
To write this loop, you use the “while
” keyword, followed by a condition wrapped in brackets (()
). JavaScript will run any code referenced in the code block ({ }
) will be run while this condition is true
.
A while
loop will only execute if the condition is true
. If you want to perform an action and then begin the loop, you can look at JavaScript’s “do while
” loop.
Below you can see the basic syntax for writing this loop within JavaScript.
while(condition) {
//Code is ran while above condition is true
}
Execution Flow of the while Loop
Let us start by exploring the execution flow of the while loop. As there isn’t a considerable amount to this type of loop, our explanation will be super simple.
To show you this workflow let us start with a simple JavaScript while loop that will print the message “Looping” to the console.
let count = 0;
while (count < 5) {
count++;
console.log("Looping");
}
With this tiny script, let us go through what is happening.
- The condition specified within the brackets (
()
) is evaluated before the loop will run.
For this example, we check whether the “count
” variable is less than5
. - If the condition is
true
, the code within thewhile
loop is run.
However, if the condition isfalse
, the loop will stop, and JavaScript will not run the code. - Our code block increases the value of the “
count
” variable by1
and prints the message “Looping
” to the console. - Once the code finishes executing, the loop returns to step 1
As you can see, there are only really three steps to the execution flow of the while loop. They are significantly more straightforward to understand than a for loop.
Using a while Loop in JavaScript
Now that you know the syntax of the while loop in JavaScript, let us explore a couple of examples of using it.
Writing a Simple while Loop
Let us start with a simple example where we will count up from 0
to 10
. This example shows you how the condition statement works for a while loop in JavaScript.
We start the script by creating a variable called “count
” using the “let
” keyword and assigning it the value of 0
.
In our next line, we start our while loop, using the condition to check if the “count
” variable is less than 10
. The while loop will continue to run until “count
” is equal to or greater than 10
.
Within the loop, we increase the value of the count
variable by one by using the ++
operator.
The last thing we do in our loop is log the value of the count
variable to the console.
let count = 0;
while (count < 10) {
count++;
console.log(count);
}
Below is the output you will get after running the JavaScript while loop.
1
2
3
4
5
6
7
8
9
10
Breaking out of a while Loop
JavaScript has a couple of keywords that you can use to control the flow of a while loop. One of these supported keywords is called “break
“.
Using this keyword, you can stop the loop even if the condition is not met. Let us take the example we wrote for “Writing a Simple while Loop
” to showcase this.
Within this while loop, we will add a conditional statement, stopping the script using the break keyword if “count
” equals 2
.
let count = 0;
while (count < 10) {
count++;
if (count == 2) {
break;
}
console.log(count);
}
Below, you can see that the break
statement stopped the loop once the count
variable was equal to 2
.
1
Skipping a while Loop Using the continue Keyword
While a while
loop is running, it is possible to skip the rest of the code block and return to the start. To control a while loop like this in JavaScript, you will need to utilize the “continue
” keyword.
With the example below, we have written a basic JavaScript while
loop that will continue to run while the “count
” variable is less than 10
.
On every loop, we increment the value of count by one by using the post-increment (++
) operator.
We use a conditional if statement to see if the modulus (%
) of 2
is not equal to 0
(This will check if the count is an even number). If this condition is met, we skip to the top of the loop by using the “continue
” keyword.
let count = 0;
while (count < 10) {
count++;
if (count % 2 != 0) {
continue;
}
console.log(count);
}
Below you can see that our use of the continue keyword ensured we only printed even numbers.
2
4
6
8
10
Looping Indefinitely using a while Loop in JavaScript
You can make a while loop in JavaScript continue indefinitely by setting the condition to something always considered true. Of course, the easiest way to do this is to use the Boolean value “true
“.
With our example below, we use a while
loop, setting the condition to true
. Within this loop, we log the message “Always Looping
“.
Since we are creating an infinite loop, the only way to stop it is to either use “break
” within the loop or stop the script entirely.
while (true) {
console.log("Always Looping");
}
Below, you can see the output from running this script. You will continue to see this message logged till you stop the script.
Always Looping
Conclusion
Hopefully, you now have a good understanding of how to write and use a while loop in JavaScript.
A while loop allows you to continually run the same code block while a condition is true.
If you have any questions about using a while loop, please comment below.
Be sure to check out our other JavaScript tutorials and our many other coding guides.