This tutorial will focus on string operators supported by the JavaScript language.
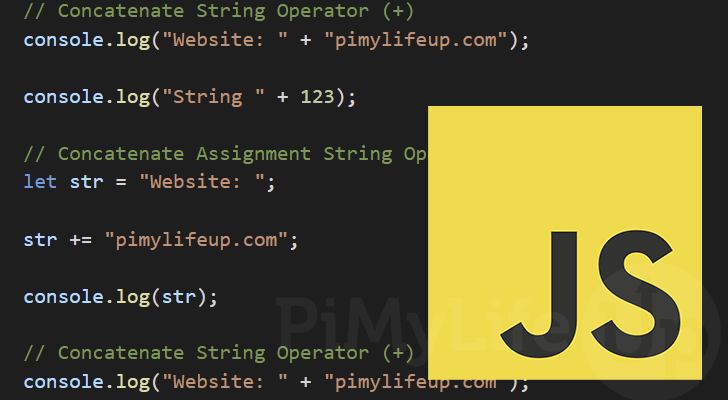
A string operator in JavaScript, simply put, is an operator that operates on a string.
There are only two operators that JavaScript supports for modifying a string. These operators allow you to join one string to another easily. These operators are called “concatenate” and “concatenate assignment“.
These two operators allow you to join one string to another.
- Concatenate: This refers to appending one string to another.
- Concatenate Assignment: Similar to the previous but refers more specifically to appending a string to the end of a variable. Assigning the final result to that variable.
You will be familiar with the symbols used in these two operators as they are used in both JavaScript assignment operators and arithmetic operators.
To showcase JavaScript’s string operators, we have created a small table. This table shows both the supported string operators, their names, an example of them being used, and the result of that operation.
Operator | Name | Example | Result |
---|---|---|---|
+ | Concatenate | “str1” + “str2” | String are joined. Results in “str1str2” |
+= | Concatenate Assignment | str += “str2” | String is appended. str has “str2” appended to it. |
Please note that JavaScript’s string operators will attempt to convert non-strings to a string. The data type that you include must have some way of being converted. For example, JavaScript can easily convert the number 112
to a string.
Example of String Operators in JavaScript
Over the following examples, we will explore the string operator in JavaScript.
These examples will give you a rough explanation of the operator and an example of how it is used within actual code.
Concatenate String Operator (+
) in JavaScript
The concatenate operator (+
) is a useful string operator in JavaScript that lets you join one string to another. It uses the plus symbol just like the addition arithmetic operator but works drastically different underneath the hood.
To use this operator, you must specify a string on the left and right sides of the concatenate operator (+
).
a + b
Copy
If you pass in a number on either side, JavaScript will convert it to a string before concatenating the values.
You can also join as many strings together as you would like. Just be wary of memory constraints.
Example of using the Concatenate String Operator
Let us write two quick examples to show you how the concatenate string operator (+
) joins strings in JavaScript.
- With the first example we are joining two strings together. This is the easiest usage of the operator for JavaScript to handle.
- For our second example, we use a string and a number as the two values we want to concatenate.
JavaScript will convert our number to a string before joining it with our left operand.
console.log("Website: " + "pimylifeup.com");
console.log("String " + 123);
Copy
The results below show how JavaScript handled the joining of strings using the concatenate string operator.
Website: pimylifeup.com
string 123
Concatenate Assignment String Operator (+=
)
In JavaScript, the concatenate string assignment operator (+=
) allows you to append a string to the end of another string easily. This is useful when you want to add a string onto a string contained within a variable.
This operator is used by specifying a variable, followed by the plus (+
) and equals (=
) sign, then the string you want to be appended to the variable.
var += a
Copy
Example of using the Concatenate Assignment String Operator
The example below will explore how you can use this string operator in JavaScript.
- We start this example by declaring a variable called “
str
” and assigning it the string “Website:
“. This is the variable we will append a string to. - On the following line, we use the concatenate assignment operator to append the text “
pimylifeup.com
” to the end of our “str
” variable. - We finish the example by using the “
console.log()
” function to log the result to the console.
let str = "Website: ";
str += "pimylifeup.com";
console.log(str);
Copy
With the output below, you can see the result of this string operator in JavaScript.
Website: pimylifeup.com
Conclusion
At this point, you should now have a good idea of how to use string operators in JavaScript.
These operators allow you to join one string to another with relative ease.
Please comment below if you have any questions about using JavaScript’s concatenate or concatenate assignment string operators.
To learn more, browse our JavaScript tutorials section. Alternatively, browse all of our coding guides to learn new languages.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support