In this tutorial, we will show you how to utilize the continue statement in JavaScript.
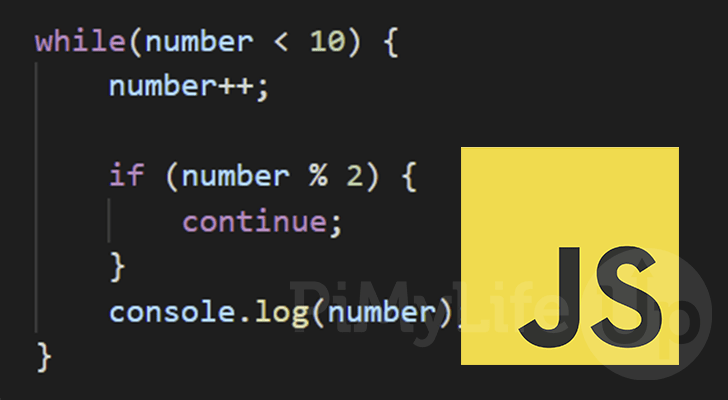
The continue statement in JavaScript is one of the various ways that you can control the flow of loops.
This statement allows you to skip the current iteration of the loop. This feature is useful when you have bits of code that you only want to be executed when certain conditions are met.
This statement is compatible with all loop types supported within JavaScript. This includes the for, for..of, for…in, while, and do…while loops.
Over the next section, we will show you how to use the continue statement within JavaScript.
Syntax of the continue Statement in JavaScript
Before we proceed any further, we can check out the syntax of the continue statement within JavaScript. As a statement, it is very simple to use.
Below, you can see the continue statement alongside its optional parameter “LABEL
“.
continue [LABEL];
When used without the label, the continue statement will perform the following action when utilized within a loop.
- When used in a while loop, the continue statement will jump the loop back to the conditional check.
- In a for loop, the continue statement will jump the loop to the update expression.
However, if you utilize the optional label, the continue statement will instead jump to that label. You must only apply these labels to loops.
Additionally, the continue statement can only jump to a label that it has been nested within. We will explain this concept further later on in this tutorial.
Typically you will use this keyword within JavaScript’s conditional if statement like we have shown below. This way, JavaScript will only skip the current iteration if that condition is met.
if (condition) {
continue [LABEL];
}
Examples of Using JavaScript’s continue Statement
In this section, let us explore using JavaScript’s continue statement in both the for and while loops.
We won’t be covering the “do...while
“, “for...of
” or “for...in
” loops since this functionality will work the same.
You will quickly see how straightforward it is to use the continue statement within your code.
Using continue with a while Loop in JavaScript
For this first example, we will show you how JavaScript’s continue statement works within a while loop.
With our example, we will increment a number on every loop and only print the value when it is even.
At the top of the code example, we define a variable with the name “number
” and assign it the value of 0
.
We then create our while loop with the simple condition to keep running while “number
” is less than (<
) 10
. On every loop, the “number
” variables value will be increased by 1
.
After the variable is incremented, we have a simple if statement that checks if “number
” is not evenly divided by 2.
If the modulus returns 1 (Is an odd number), the continue statement will be fired, and the loop will jump to the beginning.
However, if the modulus returns 0 (Is an even number), the continue statement won’t run, and we will log the value to the console.
let number = 0;
while(number < 10) {
number++;
if (number % 2) {
continue;
}
console.log(number);
}
After running the example above, you should end up with the following output. Since we used JavaScript’s continue statement, you can see that JavaScript skipped every odd number.
2
4
6
8
10
How to use the continue Statement with a for Loop
For the second example, we will explore how JavaScript’s continue statement works when used within a for loop. It is essentially the same as a while loop, but we will explore it still.
Within this example, we will write a simple for loop that will increment our “i
” variable from 0
to 10
.
We utilize an if statement within the loop to check if the current number is even. If the number is even, we use the “continue
” statement to jump to the update part of the loop.
If the number is odd, then the code will continue to run and the value will be printed to the console by using “console.log()
“.
for (let i = 0; i < 10; i++) {
if (i % 2 === 0) {
continue;
}
console.log(i);
}
The example code above will have produced the following output within the console.
1
3
5
7
9
Using the Label of the continue Statement in JavaScript
Now that you can see the continue statement’s basic usage, we can now explore how to use its label functionality.
By using labels, you can make JavaScript’s continue statement jump to an outer loop. This is useful when you have two loops nested and want to jump from the nested loop to the outer one.
To apply a label to a loop, you need to use a word, followed by a colon (:
), then the loop. You can then jump to this part of the loop by referencing the word you used to label the loop.
An example of how labeling a loop and jumping to that label is shown below.
example: for (let number = 0; number < 10; number++) {
//Do some stuff here
continue example;
}
Example of using the continue Statement with a Label
We can now write a simple example that will use two nested for loops. The outermost loop will be given the label “outer”.
Both of these for loops will count from 1 to 5. But within the nested loop, we have a conditional statement that checks if “x
” + “y
” is identical to 5.
If these added up values are identical to 5, then JavaScript will run our continue statement jumping the script back to the outer loop. It jumps to this loop because we labeled it “outer
” and referenced that next to our statement.
outer: for (let x = 1; x < 5; x++) {
for (let y = 1; y < 5; y++) {
if ( (x + y) === 5) {
continue outer;
}
console.log(x, y);
}
}
After running this script, you will end up with the following result. With this, you can see that whenever the combined value was equal to 5, JavaScript jumped to the outer loop.
1 1
1 2
1 3
2 1
2 2
3 1
Conclusion
Hopefully, at this point, you will now have a good understanding of how to use the continue statement in JavaScript.
This statement helps you control the flow of the various loops supported within this language.
If you have any questions about using the continue statement, please comment below.
You can also check out our many other JavaScript tutorials or if you want to learn a new language, view our coding guides.