In this tutorial, you will learn how to use the typeof operator within JavaScript.
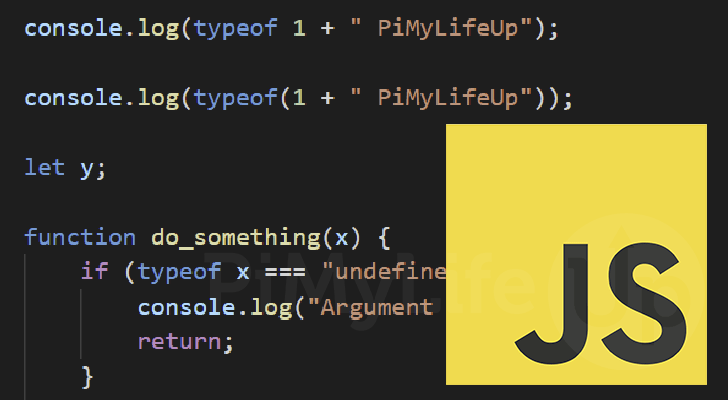
In JavaScript, the typeof operator allows you to easily get the specified variable type.
This operator is essential in JavaScript as a variable is dynamically typed.
By using typeof, you can use JavaScript to return the data type as a string. You can then use this string to check whether the variable is in the expected type.
For example, you can use typeof within a function where you need to perform a different action depending on the type of variable passed in.
Over the following few sections, we will show you the syntax of the JavaScript typeof operator and how to use it within your code.
Syntax of the typeof Operator in JavaScript
The typeof operator has a straightforward syntax within JavaScript as it is a unary operator that works on a single variable.
All you need to do is write “typeof
” followed by an operand. Typically this operand will be a variable name or a value.
Written this way, the typeof operator will get the variable type of the immediate operand only.
typeof OPERAND
You can also write the typeof operator by utilizing brackets. This is useful when you want to get the type from the result of an operation.
For example, if you added a number to a string using the concatenation operator and used the typeof operator without the brackets, you would get "NUMBER"
as the type instead of "STRING"
.
This is because without the brackets, it only operates on the immediate operand. Using brackets makes it consider the whole expression.
typeof(OPERAND)
The operand is an expression that represents an object or primitive data type. A primitive data type is a base variable type such as a string, number, Boolean, etc.
The typeof operator will return the data type of the operand as a string.
Data Returned by the typeof Operator
With the table below, you can quickly see the strings that the typeof operator in JavaScript returns when used on a particular data type.
You can also check the return result of JavaSript’s typeof operator by using the “console.log()
” function.
Data Type | Result |
---|---|
Number | "number" |
BigInt | "bigint" |
Boolean | "boolean" |
String | "string" |
Symbol | "symbol" |
Function | "function" |
Undefined | "undefined" |
Null | "object" |
Any Other Object | "object" |
Using the typeof Operator in JavaScript
Now that we know how the typeof operator is written within JavaScript let us explore using it within some actual code.
The following examples will show various ways you can utilize this operator, as well as the values returned.
Before continuing, we must note that you should always use the identical operator (===
) when comparing the result from the typeof operator.
The Two Different Ways of Using the typeof Operator
As mentioned earlier in this guide, there are two ways that you can utilize the typeof operator in JavaScript. One is to use brackets. The other is to use it without any brackets.
The behavior is almost the same except when used on an operation. When used without the brackets, the typeof operator will only return the data type of the value directly after it.
To showcase this, we will use the addition operator to add a number to a string and use the typeof operator to return the data type.
With our examples here, we are simply using the number 1
and adding the string " PiMyLifeUp"
to it. So, with one example, we will use typeof without the brackets, the other, we will use brackets.
Additionally, we use “console.log()
” to output the final data type for each operation.
console.log(typeof 1 + " PiMyLifeUp");
console.log(typeof(1 + " PiMyLifeUp"));
With this result, you can see that when using typeof without any brackets it only returned the data type of the immediate variable.
However, using brackets, the data type of the enter expression was returned by JavaScript’s typeof operator.
number PiMyLifeUp
​string
Using typeof to Check if the Passed in Variable is Defined
This example shows you how you can use the typeof operator to check if a variable is undefined.
We start this example by declaring the variable “y
” but not assigning it a value, leaving it undefined.
After this, we define a simple function called “do_something()
” that takes a single parameter.
- Within this function, we use JavaScript’s typeof operator to see if the passed-in value’s data type is identical (
===
) to"undefined".
If the data type of the “x
” variable is undefined, then the text “Argument x is undefined
” will be logged to the console.
We use “return;
” to stop the execution of the function.
For our last part, we make two calls to our new function. The first call passed in the variable (y
) we created but left “undefined
“.
The second call to the function passes in the string "Defined value"
.
let y;
function do_something(x) {
if (typeof x === "undefined") {
console.log("Argument x is undefined");
return;
}
console.log(x);
}
do_something(y)
do_something("PiMyLifeUp");
After running the JavaScript above, you should have the following two strings printed out.
You can see with the result that the “typeof
” operator returned our “x
” variable’s type as "undefined"
, and the string “Argument x is undefined
” was logged to the console.
Since our second value was a string, the “typeof
” operator would have returned “string
“, meaning the string "PimyLifeUp"
was printed to the console.
Argument x is undefined
PiMyLifeUp
Understanding JavaScript’s typeof Operator When Used on null
One oddity of the typeof operator in JavaScript is the way it handles a “null
” value. Instead of returning a data type as “null
“, the operator returns “object
“.
This behavior comes from the very early days of JavaScript. Unfortunately, since this behavior has been around for so long, it is tough to change this without potentially breaking years of scripts.
You can see this for yourself by using the following line of JavaScript.
console.log(typeof null);
Below you can see how the typeof operator returned “object
” for our “null
” value.
object
NaN is Considered a Number by JavaScript’s typeof Operator
When you use the typeof operator in JavaScript to check if a data type is a number, you might notice that it considers “NaN
” to be a “number
“.
You can check this behavior by looking at the following example. We intentionally declare a variable called “number
” and set it to the NaN
variable type.
Next, we have a simple JavaScript conditional if statement. We use this statement to check if the “number
” variables type is identical to "number"
.
let number = NaN;
if (typeof number === "number") {
console.log("This will still run");
}
If you need to work around this behavior, you can utilize the logical AND and logical NOT operators alongside the the “isNaN()
” function
let number = NaN;
if (typeof number ==="number" && !isNaN(number)) {
console.log("This will no longer run")
}
With these changes to the script, you shouldn’t have any text printed to the console, as the “isNaN()
” function will catch any variable that is not a number.
Conclusion
Throughout this guide, we have shown you how to use the typeof operator within JavaScript.
This operator gets the data type of the specified expression as a string. This is useful for ensuring that you deal with the correct data type.
Please comment below if you have any questions about using this operator.
Check out our many other JavaScript tutorials to learn more about the programming language. If you want to learn another language, we have various other coding tutorials.