This tutorial will show you how to use the delete operator in JavaScript.
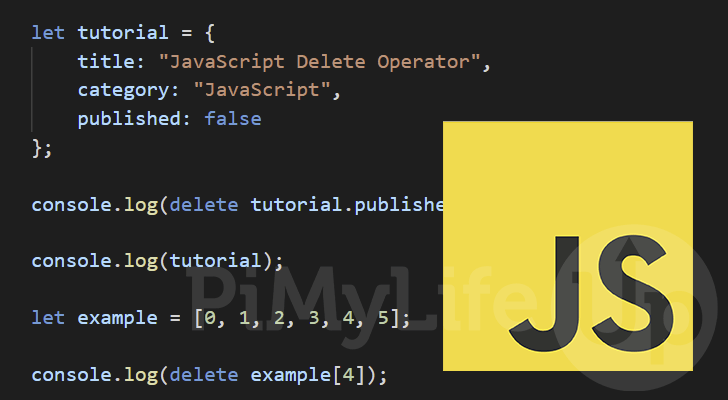
The delete operator is used in JavaScript to remove a property from an object. A property is a part of an object with a name and a value. It will not work on a variable or function.
Once you delete a property, you will no longer be able to access it until it has been added to the object. Accessing a deleted element will returned “undefined
“.
In JavaScript, the delete operator doesn’t help with freeing memory directly. Instead, memory management is handled automatically by the underlying garbage collector. When an object’s property is devoid of references, the garbage collector will eventually clear it.
You should never use the JavaScript delete operator on inbuilt objects such as window, Math, and Date. Using delete on any of these will likely cause your web browser or application to crash.
Over the following sections, we will show you how to use the delete operator within JavaScript.
Syntax of the delete Operator in JavaScript
Let us start by exploring the syntax of the delete operator within JavaScript. This operator is very straightforward to use but is also quite dangerous.
There are two ways you can use this operator. Both behave the same and are just different ways you can reference an object’s property.
With the first method, you use the “delete
” keyword, followed by the object name, a full stop (.
), then the property you want to delete.
delete object.property;
You can also delete an object property like you would reference an element of an array.
You start with JavaScript’s “delete
” keyword, followed by the object name, two square brackets ([ ]
), then the property name within the square brackets.
delete object[property];
If JavaScript successfully deletes the object property, JavaScript will return true
. Otherwise, if it failed, false
.
However, if JavaScript is in strict mode and it failed to delete due to the property being unwritable, JavaScript will throw an error instead of returning false.
If the object property doesn’t exist, JavaScript will still return true
.
Using JavaScript’s delete Operator
This section will show you how to use the delete operator within JavaScript. In addition, these examples will show you some of this operator’s do’s and don’ts.
Basic Usage of JavaScript’s delete Operator
The primary way to use the delete operator in JavaScript is to delete a property of an object. Most things in JavaScript are considered objects, so this has quite a bit of potential usage.
To showcase deleting an object’s property, we will create our own object called “tutorial
“. If you are unfamiliar with objects in JavaScript, they can be defined using the curly brackets ({ }
).
We assign some sample key values to this object so we have some properties that we can delete. The three properties that we add are “title
“, “category
“, and “published
“.
After the object is created, we use the delete operator to delete the “published
” property of our “tutorial
” object. We log the true
or false
result returned from the delete operation.
Finally, we use “console.log()
” to log what our object looks like after deleting the “published
” property.
let tutorial = {
title: "JavaScript Delete Operator",
category: "JavaScript",
published: false
};
console.log(delete tutorial.published);
console.log(tutorial);
After running the above example, you can see how the JavaScript delete operator removed our “published” property.
Additionally, you can see that the operator returned “true
” since the delete operation was successful.
true
{title: 'JavaScript Delete Operator', category: 'JavaScript'}
Don’t use the delete Operator on an Array
As mentioned earlier, JavaScript handles most things as objects. So, for example, you can use the delete operator on an array.
We will have to preface this by saying that you should not use the delete operator on an array as it causes some issues. Instead, you should rely on other functions such as pop()
, shift()
, or splice()
when interacting with an array in JavaScript.
TLet us start this example by creating an array to showcase some of the weird edge cases. This array will be called “example
” and hold numbers 0
through 5
.
We will then use JavaScript’s delete operator to delete the 4th element in our array. Finally, we log the result of the delete operation to the console.
Finally, we log the array itself to the console. Logging the array to the console will show us all the properties within the object and an issue delete causes.
let example = [0, 1, 2, 3, 4, 5];
console.log(delete example[4]);
console.log(example);
Below you can see the result of using the delete operator on an array. First, the delete operator returned true since the deletion was successful.
After that, however, you can see where problems occur. The 4th element of the array was deleted but left an empty spot in its place, with the length of the array still remaining the same.
This is because the delete operation doesn’t consider how the array object functions. It just mindlessly removes that specific property.
true
(6) [0, 1, 2, 3, empty, 5]
0: 0
1: 1
2: 2
3: 3
5: 5
length: 6
delete Doesn’t work on Variables or Functions
Another note about the delete operator is that it does not work on variables or functions.
We will showcase this by writing a bit of JavaScript that will use the delete operator on both a variable and a function.
At the top of our example, we create a variable called “example
” and use the JavaScript assignment operator to give it the string “pimylifeup
“.
In addition to creating a variable, we define a simple function called “foo
” that returns the string “bar
“.
After declaring both the variable and object, we use the delete operator on both of them. Finally, the result of both operations is logged to the console.
let example = "pimylifeup";
function foo() {
return "bar";
}
console.log(delete example);
console.log(delete foo);
You can see that JavaScript failed to delete either our variable or function, returning false
on both instances.
false
false
Conclusion
In this tutorial, we explained how to use the delete operator within JavaScript.
This operator is purely aimed at deleting the properties of an object and shouldn’t be used on variables, functions, or core JavaScript components.
If you have any questions about using this operator, please comment below.
We have many other JavaScript tutorials if you want to learn more about the programming language. Alternatively, if you want to learn a new language, you should check out our wealth of other coding guides.