In this tutorial, we will be showing you how to write a for…in loop in JavaScript.
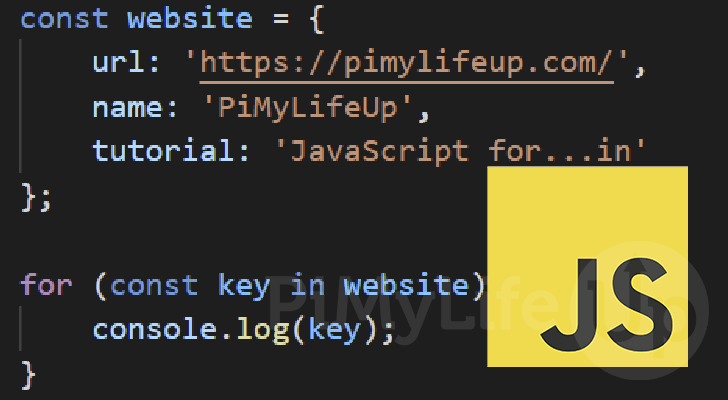
In JavaScript, the for…in loop allows you to iterate over the enumerable property names of an object easily. Sometimes these names are also referred to as the property keys.
Unlike PHP’s foreach loop, JavaScript’s for…in loop will return an object’s property names/keys and not its values.
This type of loop is useful when you want to iterate over the properties of an object quickly. However, it is not a great solution if you want to iterate over an array.
If you want to iterate over an array in JavaScript, you should instead use the for…of loop, “.forEach()
” function, or a simple for loop.
Over the following sections, we will show you how you can use the for…in loop.
Syntax of the for…in loop in JavaScript
Let us start this guide by exploring how you write a for…in loop within JavaScript.
You start the loop by utilizing the “for
” keyword. Within the brackets, you will first declare a variable that you will use to store the property name on each loop. You can use “var
“, “let
“, or “const
” to declare this variable.
Follow this up by using the “in
” keyword, then the name of the object you want to iterate over.
for (VARIABLE in OBJECT) {
//Code to be run
}
Copy
For every loop, JavaScript will iterate over the specified object. It will assign the property name for that iteration to the specified variable.
Using the for…in Loop in JavaScript
We will run you through various examples to show you how the for…in loop works within JavaScript.
These examples will also show you some of the various use cases of this loop. However, its primary usage is to loop through an object’s properties.
Iterating Over an Object with the for…in Loop in JavaScript
Let us start with one of the more straightforward ways of using a for…in loop in JavaScript. We will use the loop to iterate over the specified object.
We start this example code by creating an object called “website
” (A object is defined by the curly brackets {}
). Within this object, we create three properties with the names “url
“, “name
“, and “tutorial
“.
Next, we create our JavaScript for…in loop. We start by declaring the variable as a const
with the name “key
“. Then we use the “in
” keyword and specify “website
” as the object we want to iterate over.
In this loop, we use the console.log()
function to print the property name on every loop. Using this key you can also get the value of that property.
const website = {
url: 'https://pimylifeup.com/',
name: 'PiMyLifeUp',
tutorial: 'JavaScript for...in'
};
for (const key in website) {
console.log(key);
}
Copy
After running this JavaScript, you should end up with the following result. With this, you can see the for…in loop iterated over the object, printing each property.
url
name
tutorial
Using the for…in Loop to Iterate Over an Array with JavaScript
You can use JavaScript’s for…in loop to iterate over the keys of an array as they are considered an object.
However, before starting this example, we must note that you typically shouldn’t use a for..in loop to iterate over an array. Instead, you should use the JavaScript for loop or the for…of loop.
The reason for this is that this type of loop iterates over all enumerable elements of the array, and there are some complex rules you have to worry about to guarantee the array order.
For this example, we start the script by declaring an array called “example
” within this, we use the values "Hello"
, "World"
, "PiMyLifeUp"
, "Tutorial"
.
We then follow this up by using the for…in loop to iterate over the property names of our array. In our case, this will be the array index.
We have two usages of the console.log()
function. The first log prints the current key value, and the second uses the key to print that array value.
const example = ["Hello", "World", "PiMyLifeUp", "Tutorial"];
for (const key in example) {
console.log(key);
console.log(example[key]);
}
Copy
After running this example JavaScript code, you should end up with the following result.
0
Hello
1
World
2
PiMyLifeUp
3
Tutorial
Iterate Over a String Using the for…in Loop
For this example, we will use the for…in loop to iterate over a string. This is possible since strings are essentially handled as an array internally.
At the top of the script, we create a variable called “example_string
” and assign it the value “Hello
“.
We then use the for…in loop to iterate over the properties of the “example_string
” variable. On every loop, we print the current property name (The key) and then the letter at that position in the array.
const example_string = "Hello";
for (const key in example_string) {
console.log(key);
console.log(example_string[key]);
}
Copy
Using the above JavaScript example, you should end up with a similar result to what we have shown below.
0
H
1
e
2
l
3
l
4
o
Conclusion
Throughout this tutorial, we have shown you to write and use the for..in loop within javaScript.
This type of loop allows you to quickly and efficiently iterate over the properties of an object. However, it should typically not be used to iterate over an array.
If you have any questions about the for…in loop, please comment below.
Be sure to check out our other JavaScript tutorials, or if you want to learn a new language, our many other programming guides.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support