In this tutorial, you will learn how to use the ternary operator in JavaScript.
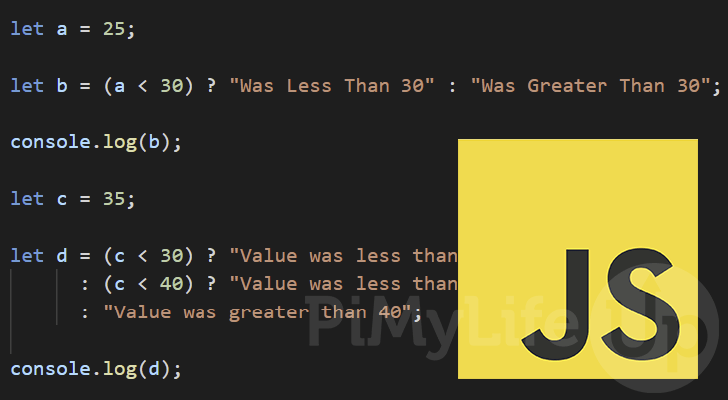
The ternary operator is an incredibly useful conditional operator supported by JavaScript. Using this operator you can return a value depending on whether a condition is true
or false
.
Out of all of the operators supported by JavaScript, the ternary operator is the only one that has three parameters.
One of the ternary operator’s best uses is simplifying your code. For example, you can use a ternary operator to set the value of a variable depending on the condition. This saves you from having to use a slightly more messy if…else statement.
Over the following few sections, we will show you how to use the ternary operatory within JavaScript.
Syntax of JavaScript’s Ternary Operator
As mentioned earlier, the ternary operator is slightly different in that it deals with three operands. These operands are the condition, the “true
” expression, then the “false
” expression.
When writing this operator you start by specifying a conditional statement, followed by a question mark (?
).
You then specify an expression to be executed if the condition is true
, followed by a colon (:
).
Finally, the ternary operator is ended with an expression that will be executed if your condition is false
.
condition ? true expression : false expression;
If you were to replicate the behavior of the ternary operator with an “if...else
” statement, your code would look like what we have shown below.
You can see that this operator cleanly cuts your code down from four to one line of code.
if (condition) {
true;
} else {
false;
}
Using the Ternary Operator in JavaScript
Now that we know how the ternary operator is written within JavaScript, let us show you how to use it.
We will be exploring the basic usage of this operator and how you can chain multiple ternary operators together.
Basic Usage of the Ternary Operator
For this example, we start by declaring a variable called “a
” and assign it the value of 25
.
We then declare a variable called “b
“. We will use the ternary operator to dictate this variables value using a simple condition.
For the condition of the JavaScript ternary operator we use the less-than operator (<
) to see if “a
” is less than 30
.
- If “
a
” is less than30
, the “b
” variable will be assigned the text “Was less than 30
“. - If “
b
” is greater than or equal to30
, the “b
” variable will be assigned the text “Was Greater Than 30
“.
The script ends with us logging the value stored in our “b
” variable to the console by using the “console.log()
” function.
let a = 25;
let b = (a < 30) ? "Was Less Than 30" : "Was Greater Than 30";
console.log(b);
After running the above code, you should end up with the following result logged to your console.
Was Less Than 30
Example Written as an if Statement
Now, if you were to write this same example in JavaScript using a conditional if statement instead of a ternary operator, it would look like what we have shown below.
Even though both lots of code produce the same result, you can see how the ternary operator allows you to write cleaner and more concise code.
let a = 25;
let b = "";
if (a < 30) {
b = "was less than 30";
} else {
b = "Was Greater Than 30";
}
console.log(b);
Chaining Ternary Operators
In JavaScript, the ternary operator also allows conditional chains where the right-side condition chains into another ternary operator.
By chaining ternary operators, you effectively write an “if ...else if ... else
” conditional chain. But, of course, you have to be careful that chaining the ternary operators doesn’t make your code less readable.
An example of how you could write this is shown below. We have broken each new part of the conditional chain onto a new line to make it easier to read. You can chain as many ternary operators together if you like.
condition1 ? value1
: condition2 ? value2
: value3
Now that you know that you can chain ternary operators in JavaScript let us show you a quick example.
With this example, we create a variable called “a
” and assign it the value 35
. We will use this variable in both of our conditions.
- We then create a variable called “
b
“, but JavaScript will assign its value via our chained ternary operators. - The first ternary operator will check if “
a
” is less than (<
)30
. If the value is less than30
, then the text “Value was less than 30
” will be assigned. - If the value equals or exceeds
30
, it will fall over to the second ternary operator. - With the second ternary operator, we check whether “
a
” is less than40
. If the value is less than, the text “Value was less than 40
” will be assigned. - If the value is greater than
40
, then the text “Value was greater than 40
” will be assigned.
JavaScript will assign the result of the chained ternary operator to our “b
” variable. We then log the “b
” variable to the console thanks to the use of the “console.log()
” function.
let a = 35;
let b = (a < 30) ? "Value was less than 30"
: (a < 40) ? "Value was less than 40"
: "Value was greater than 40";
console.log(b);
If you run the above example, you should end up with the text below in your console.
Value was less than 40
Example Written as an if Statement
Below you can see how you would write a chained ternary operator if you used an “if...else if...else
” conditional statement instead.
When chaining ternary operators together, you need to be more careful on how you write them to avoid your code readability being ruined.
let a = 35;
let b = "";
if (a < 30) {
b = "Value was less than 30";
} else if (a < 40) {
b = "Value was less than 40";
} else {
b = "Value was greater than 40";
}
console.log(b);
Conclusion
Throughout this tutorial, we have shown you how to use the ternary operator in JavaScript.
This is the only conditional operator supported by JavaScript and is useful for setting a value based on whether a condition is true or false.
Please comment below if you have any questions about using this operator.
We also have a variety of other JavaScript tutorials if you want to learn more about this language. We also have a variety of other coding guides if you want to start learning a new language.