In this guide, we will be showing you how to write and use a for…of loop in JavaScript.
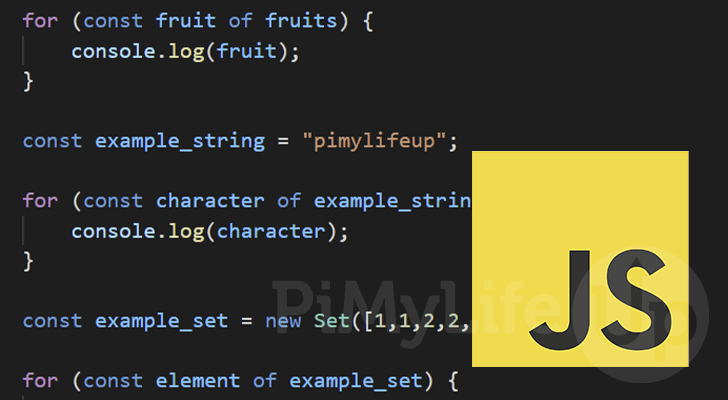
In JavaScript, the for…of loop allows you to loop over values stored within an iterable object.
These iterable objects include arrays, sets, strings, maps, NodeLists, etc. In addition, any object can implement an iterable protocol that this loop will use to process its data.
The for…of loop is a relatively new addition to the JavaScript language being implemented in ES6. However, this type of loop is supported by every major web browser.
Compared against the JavaScript for loop and for…in loop it is best used to iterate over values stored in objects such as arrays.
Over the next couple of sections, we will show you how to write and use the for…in loop within JavaScript.
Syntax of the for…of Loop in JavaScript
Let us explore how you write the for…in loop within the JavaScript language.
This loop is always started by specifying the “for
” keyword followed by two brackets (( )
). Within these brackets, you will need to specify the following data.
- First, you must specify a variable where the value will be stored for the current loop. Then, every time the loop iterates, the value of this variable will be updated.
You can declare this variable using the “var
“, “let
” or “const
” keywords. - Next, to define this as a for… in loop, you will need to use the “
of
” keyword.
Writing “of
“, tells the JavaScript compiler how it needs to handle iterating over the specified object. - Finally, the last element needed to be included in the brackets is the object you want to iterate over.
As long as the object supports the iterable protocol, this will loop over it.
Below you can see how a basic for…in loop is written within JavaScript.
for (ELEMENT of ITERABLE) {
//Code to be Ran on Every Loop
}
Examples of using for…of Loops in JavaScript
Now that we have shown you the syntax of the for…in loop, let us explore how to use it in JavaScript.
Over the following sections, we will explore several different ways of utilizing this type of loop.
Iterating over an Array using the for…of Loop in JavaScript
One of the best usages of JavaScript’s for…of loop is to iterate over an array. It makes iterating through an array incredibly straightforward.
For this example, we start by creating a constant (const
) variable called “fruits
“. Within this variable, we will store an array containing the following strings: "Apple"
, "Banana"
and "Cantaloupe"
.
Next, we create our for…of loop. Here we use “fruit
” as the variable that will contain that current loops value. If you want to adjust the value, you will need to define the variable using “let
” instead of “const
“.
Then we use the “of
” keyword followed by the array we want to iterate over, which in our case is “fruits
“.
On every loop, we use “console.log()
” to log the value that is currently in the “fruit
” variable.
const fruits = ["Apple", "Banana", "Canataloupe"];
for (const fruit of fruits) {
console.log(fruit);
}
After running the above example, you can see how JavaScript for…of loop iterated through each of the three values.
Apple
Banana
Cnataloupe
Using a for…of Loop on Strings
The for…of loop can be used to iterate over a string since they are an iterable object within JavaScript.
This example, will show you how you can utilize the for…of loop to loop over a string. It is basically the same as you would handle an array.
We start this example off by declaring a string called “pimylifeup
” and assigning it to a variable called “example_string
“. As we don’t plan to modify this string, we declare it using “const
“.
Here we use the for…of loop to iterate over the “example_string
” variable. JavaScript will assign a character from this string to our “character
” variable on each loop.
To showcase JavaScript looping over your string, we use the “console.log()
” function to output the value on every loop.
const example_string = "pimylifeup";
for (const character of example_string) {
console.log(character);
}
When you run the above JavaScript example, you should end up with an output that looks like what we have shown below.
p
i
m
y
l
i
f
e
u
p
Using a for…of Loop to iterate over a Set
A set is another object that can be iterated over within the JavaScript language using the for…of loop. A “set
” is an object that ensures only unique elements exist.
Start the example by creating a variable called “example_set
” and assigning it a new set with the data [1,1,2,2,3,3]
.
Next, we use a for…of loop to iterate over our “example_set
“. Finally, we use “console.log()
” to output each element within the set on every loop.
const example_set = new Set([1,1,2,2,3,3]);
for (const element of example_set) {
console.log(element);
}
From the above example, you should end up with the following result printed on the command line. Since this is a set, only unique entries will be output.
1
2
3
Iterate over a Map using a for…of Loop in JavaScript
The for…of loop can also be used to iterate over a JavaScript map. The map object holds key-value pairs, so you will need to handle this slightly differently by using JavaScript’s destructuring functionality.
Start this code example by declaring a map with some simple example data as we have below.
We will be using two for…of loops within this example to show you how you can get the data structured and destructed.
With the first loop, we are iterating over the “example_map
” variable without destructuring the data. This means on every loop you will get the mapped data as a whole and not as a separate key and value.
Our second loop changes this by using JavaScript’s ability to unpack a data structure. By using the square brackets ([ ]
) and specifying our “key
” and “value
” JavaScript will unpack the map into these two values.
For both of our loops we will log all of the values we retrieve in that loop
const example_map = new Map([['a', 'apple'], ['b', 'banana']]);
for (const entry of example_map) {
console.log(entry);
}
for (const [key, value] of example_map) {
console.log(key + ' => ' + value);
}
With the output below, you can see the two different data types produced by the two for…of loops.
["a", "apple"]
[
"b",
"banana"
]
a => apple
b => banana
How to use a for…of loop on Generators
Within JavaScript, generators are iterable objects, so you can utilize a for…of loop to iterate over them.
For this example, we will write an incredibly straightforward generator called “sampleGenerator()
“. This is defined by using “function
“, followed immediately by an asterisks (*
) then the function name.
Within this, we will simply yield 1, 2, and 3. These values will be printed out when we iterate over the generator.
We use the for…of loop to iterate over this generator. Using o as our variable name, then using a call to the generator as the “iterable object”.
On every loop, the “console.log()
” function is used to output the current value.
function* sampleGenerator() {
yield 1;
yield 2;
yield 3;
};
for (const o of sampleGenerator()) {
console.log(o);
}
Below you can see the output that we retrieved from looping over our “sampleGenerator()
“.
1
2
3
Conclusion
Within this tutorial, we have shown you how to use the for…of loop in JavaScript. It is a powerful loop that is useful whenever you need to iterate over data.
This loop is one of the best ways of iterating over the values stored within an array.
If you have any questions about using the for…of loop, please comment below.
Be sure to check out our many other JavaScript tutorials and our other coding guides.