In this Raspberry Pi humidity sensor tutorial, we will show you how to connect the DHT22 sensor to the Raspberry Pi and how you can use Python to read data from the sensor.
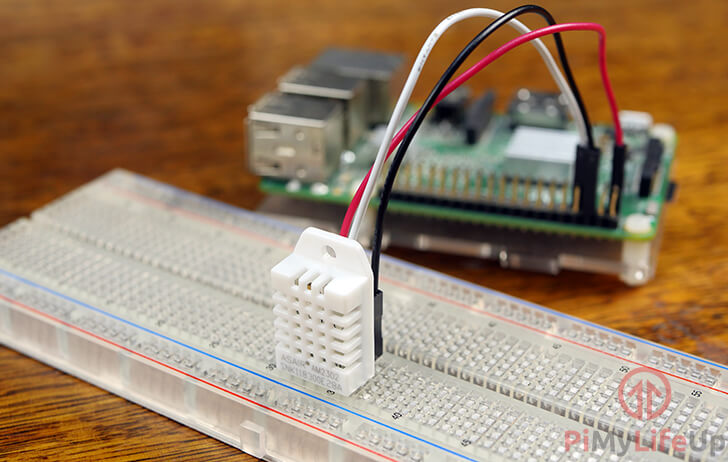
The DHT22 is a versatile and low-cost humidity sensor that can also calculate the temperature of an area.
This sensor has a relatively long transmission distance, allowing the sensor to transmit data through wires up to 20m away from the Raspberry Pi.
As a bonus, the DHT22 is a digital sensor with an inbuilt analog to digital converter. The converter makes it a lot easier to connect the sensor to the Raspberry Pi as you do not need to deal with any additional chips.
The biggest downside to the DHT11 and DHT22 sensors is that they are quite slow sensors. They have a sampling rate of once every second for the DHT11 and once every 2 seconds for the DHT22.
You can also use the DHT22 with the Arduino, so be sure to check that tutorial if you would rather use an Arduino board.
While this tutorial covers connecting the DHT22 to the Raspberry Pi, it will also work with the DHT11 and AM2302 humidity sensors as they all use the same pinouts.
Equipment
Below is all the equipment that you will need for connect the DHT22 Humidity Sensor to your Raspberry Pi.
Recommended
- Raspberry Pi Amazon
- 8GB Micro SD Card Amazon
- Breadboard wire Amazon
- DHT22 Humidity Sensor Amazon
- 10k ohm resistor Amazon (Brown, Black, Orange, Gold)
Optional
- Raspberry Pi Case Amazon
Video
The video below will take you through the steps to assembling the humidity circuit and how to connect it to your Raspberry Pi. It also covers the steps on how to write a simple Python script to talk with the DHT22 sensor.
If you prefer a written tutorial. then you can find that right underneath the video.
Raspberry Pi Humidity Sensor Hardware Setup
In this section of the tutorial, we will show you the process on how to connect your DHT22 humidity sensor to the Raspberry Pi.
Thanks to the DHT22 being a digital sensor, it is incredibly straightforward to connect to the Raspberry Pi. The single data pin is able to connect directly to the Raspberry Pi’s GPIO pins.
You can follow our guide below to see how to connect the DHT22 to your Raspberry Pi.
DHT22 Pinout
To make things easier when assembling the humidity sensor circuit we have included the pinout of the DHT22 sensor.
This diagram should help you work out where each pin needs to go on the Raspberry Pi.
- Pin 1 is VCC (Power Supply)
- Pin 2 is DATA (The data signal)
- Pin 3 is NULL (Do not connect)
- Pin 4 is GND (Ground)
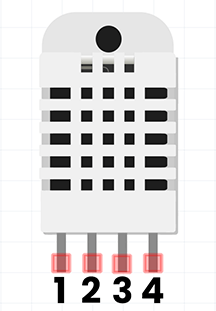
Raspberry Pi DHT22 Circuit
Here we have included a couple of ways you can put the Raspberry Pi Humidity sensor circuit together.
You can either rely on our two diagrams below to see what pins need to go to what or use our written steps below.
- Place a 10k resistor between Pin 1 and Pin 2 of the DHT22
- Wire Pin 1 of the DHT22 to Physical Pin 1 (3v3) on the Pi
- Wire Pin 2 of the DHT22 to Physical Pin 7 (GPIO4) on the Pi
- Wire Pin 4 of the DHT22 to Physical Pin 6 (GND) on the Pi
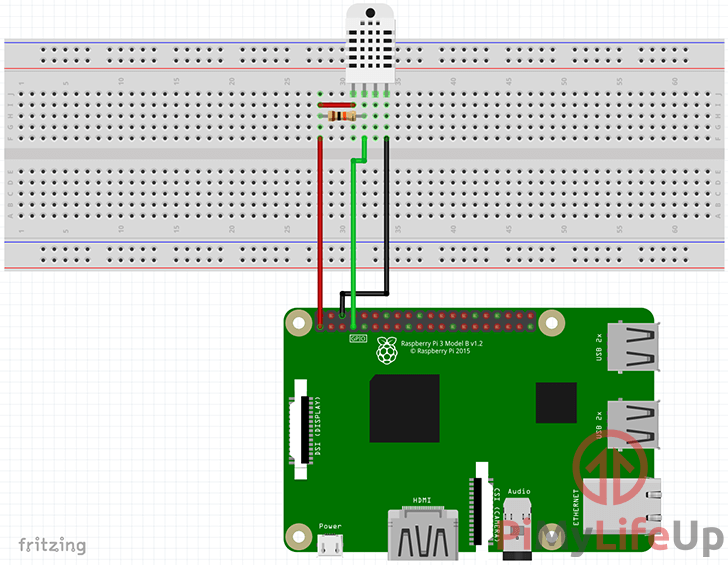
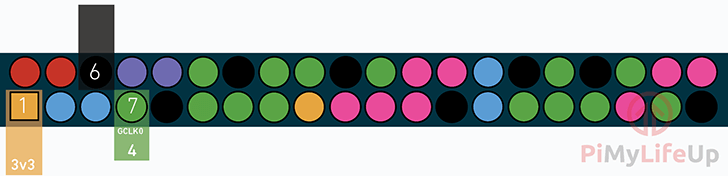
Preparing the Raspberry Pi to talk with the Humidity Sensor
In this section we will be preparing your Raspberry Pi to talk with the DHT22 humidity sensor.
Mainly we need to install Python 3 to the Pi and also set up a Python Virtual Environment.
Setting up your Raspberry Pi
1. Before we get started with programming a script for the Raspberry Pi humidity sensor, we must first ensure that we have the latest updates on our Raspberry Pi.
We can do this by running the following two commands to update both the package list and installed packages.
sudo apt update
sudo apt upgrade
Copy
2. With our package list now up to date, we need to go ahead and install both python 3 and pip. We will be using both of these packages to interact with our humidity sensor.
Install both “python3-dev
” and “python3-pip
” by running the command below.
sudo apt install python3-dev python3-pip
Copy
Setting up a Python Virtual Environment for the DHT22 Humidity Sensor
3. Our first step is to create a directory where we will store the Python virtual environment for the DHT22 humidity sensor.
You can create a directory called “dht22
” in your users home directory by running the following command.
mkdir ~/dht22
Copy
4. After creating the directory, you must now change to it by using the cd command.
cd ~/dht22
Copy
5. We now need to use Python to generate the virtual environment. All of the libraries that we will be using will be installed to this environment.
Generate the Python virtual environment by typing in the following command.
python3 -m venv env
Copy
6. Once the virtual environment has been generated, we will need to tell the terminal to utilize it as its source.
You will need to run this command every time you want to interact with your DHT22 humidity sensor script. The reason for this is the Python library we are using will be installed only to this environment.
source env/bin/activate
Copy
7. Now using pip, we will go ahead and install Adafruit’s DHT library to the Raspberry Pi.
We will be using this Python library to interact with our DHT22 Humidity/Temperature sensor.
As a bonus, the library also supports the DHT11 and AM2302 humidity/temperature sensors making it a great library to learn how to utilize.
Run the following command to install the DHT library to your Raspberry Pi.
python3 -m pip install adafruit-circuitpython-dht
Copy
Programming for the Raspberry Pi humidity sensor
1. Now that we have installed the Adafruit DHT library to our Raspberry Pi we can now proceed to program with it.
First, we must make a Python script in which we will store all our code. To begin writing this file, you can enter the following command into your Raspberry Pi.
nano ~/dht22/humidity.py
Copy
2. Within this file, we need to enter the following lines of code. We will explain each section of the code as we go along.
a. The first library we will be importing in this script allows us to sleep the script between readings. This stops the script from constantly reading from the sensor when it doesn’t need to.
import time
Copy
b. This line imports the “Adafruit_DHT
” library that we obtained using pip
in the previous section.
We will be using this library to talk and interact with the DHT22 sensor. The library allows us to very easily retrieve the temperature and humidity from the sensor with a few lines of python code.
import adafruit_dht
Copy
c. The Adafruit DHT22 Library also required us to import the “board” library.
This library is used internally by Adafruit for selecting the pins your humidity sensor is attached to.
import board
Copy
d. Our next step is to create our “dht_device
” variable that points to Adafruit DHT22 library. Into this function call we pass in the pin our DHT22 sensor is connected to on our Raspberry Pi.
We use this object to get the current temperature and humidity from the sensor.
Since we are using the GPIO4 pin we need to use the “D4
” option from the board library.
dht_device = adafruit_dht.DHT22(board.D4)
Copy
e. Since we want our Python script to constantly get readings from the humidity sensor we will need to start an infinite loop. This loop makes the script continually run until you terminate it.
All code that is within this loop must be indented.
while True:
Copy
f. One problem with the DHT22 library is that it can commonly throw errors. This isn’t necesserally a problem with the DHT22 library or your Raspberry Pi but more with how the sensor itself works.
To prevent an error from stopping the script we must run our code within a “try
” block.
Indent the code by another four spaces after this block.
try:
Copy
g. Finally within our try block we can finally get our first temperature value from our sensor. Reading a value from the sensor is simple as grabbing the “temperature” value from the “dht_device
” object.
Since the DHT22 reports temperature in Celsius we will store the value in a variable called “temperature_c
“.
temperature_c = dht_device.temperature
Copy
h. While the DHT22 humidity sensor might not give us the value in Fahrenheit, luckily it is very easy to convert the value.
The following line of code converts our Celsius temperature to Fahrenheit and stores it in a variable called “temperature_f
“.
temperature_f = temperature_c * (9 / 5) + 32
Copy
i. Luckily, the humidity reading is super simple and is returned as a percentage that we store within the “humidity
” variable.
humidity = dht_device.humidity
Copy
j. Finally, once we have gotten the temperature and humidity values from our DHT22 sensor we can print the to the terminal by making use of the “print()
” function.
print("Temp:{:.1f} C / {:.1f} C Humidity: {}%".format(temperature_c, temperature_f, humidity)
Copy
k. Now that we are finished with our try block we must end it with the following block of code.
This code catches any errors that might be caused by the DHT22 library on our Raspberry Pi and prints the error to the terminal. By catching the error the script will continue to run.
except RuntimeError as error:
print(err.args[0])
Copy
l. Finally, we end every loop by running a short 2 second sleep of the script. This gives us time between recordings and reduces the chance of running into errors.
time.sleep(2.0)
Copy
3. Once you have finished entering the code, it should look like what we have displayed below.
import time
import adafruit_dht
import board
dht_device = adafruit_dht.DHT22(board.D4)
while True:
try:
temperature_c = dht_device.temperature
temperature_f = temperature_c * (9 / 5) + 32
humidity = dht_device.humidity
print("Temp:{:.1f} C / {:.1f} F Humidity: {}%".format(temperature_c, temperature_f, humidity))
except RuntimeError as err:
print(err.args[0])
time.sleep(2.0)
Copy
4. When you are happy that all the code you entered is correct you can then save the file by pressing CTRL + X, then Y followed by ENTER.
5. With the script now saved, let’s go ahead and run it so that we can start to see the data from the humidity sensor.
You can run this script by running the following command.
python3 ~/dht22/humidity.py
Copy
6. From this command, you should start seeing results like what we have below. The temperature and the humidity should be displayed through the command line.
Temp:24.0 C / 75.2 F Humidity: 47%
Temp:24.0 C / 75.2 F Humidity: 48%
Temp:24.0 C / 75.2 F Humidity: 49%
Temp:24.0 C / 75.2 F Humidity: 52%
7. In the next section of the tutorial, we will show you how you can modify this script so that it logs the data to a file. The file will be able to be read by a program such as Microsoft Excel or Google Spreadsheets.
Logging the Data from the Raspberry Pi Humidity Sensor
1. Let’s start by creating a new script. This script will be somewhat similar to our previous script but with a few changes made so that we can write to a file.
Start by running the following command to begin writing our new script.
nano ~/dht22/humidity_logger.py
Copy
2. Within this file enter the following lines. We will only be explaining the new additions to the script in this section.
a. Here we add the import for both the “os
” and “time
” libraries. We utilize the “os
” library so we can check if our CSV file exists before we try to write to it.
We use the “time
” library so that we can timestamp each new row with the current date and time.
import os
import time
import adafruit_dht
import board
Copy
b. There is no difference with this line, keep it as you had in the previous script.
dht_device = adafruit_dht.DHT22(board.D4)
Copy
c. Here we wrap everything within a try statement to handle any potential errors.
We open up a handle to our “/home/pi/humidity.csv
” file with the “a+
” tag applied. The a+ tag means that any data written to the file will be appended to the end of it.
Next, we use the “os
” library to see if we have ever written to this file before. If the size returns as 0 we then write an initial line to the file. This line will contain our column headers so you can understand the data easier.
try:
f = open('/home/pi/humidity.csv', 'a+')
if os.stat('/home/pi/humidity.csv').st_size == 0:
f.write('Date,Time,Temperature C, Temperature F,Humidity\r\n')
except:
pass
Copy
d. Here we have our while loop again. We utilize the DHT Library to read data from our DHT22 humidity sensor again.
This function will store the data it reads from the sensors into our “humidity
” and “temperature
” variables.
while True:
temperature_c = dht_device.temperature
temperature_f = temperature_c * (9 / 5) + 32
humidity = dht_device.humidity
Copy
e. Our big change to this section of code is that instead of printing the temperature and humidity to the console, we write it to our “humidity.csv
” file. We do this by using the file handle we opened earlier.
We format the text written to the file to include both the current date, current time, our formatted temperature and humidity.
At the end of the loop, we sleep the script for 30 seconds, if you want to make it sleep longer or poll more often, then modify this number.
f.write('{0},{1},{2:0.1f}*C,{2:0.1f}*F,{3:0.1f}%\r\n'.format(time.strftime('%m/%d/%y'), time.strftime('%H:%M'), temperature_c, temperature_f, humidity))
except RuntimeError as err:
print(err.args[0])
time.sleep(30)
Copy
3. Once you have finished entering the code, it should look like what we have displayed below.
Ensure that you replace “pi
” with your own username.
import os
import time
import adafruit_dht
import board
dht_device = adafruit_dht.DHT22(board.D4)
try:
f = open('/home/pi/dht22/humidity.csv', 'a+')
if os.stat('/home/pi/dht22/humidity.csv').st_size == 0:
f.write('Date,Time,Temperature C, Temperature F,Humidity\r\n')
except:
pass
while True:
try:
temperature_c = dht_device.temperature
temperature_f = temperature_c * (9 / 5) + 32
humidity = dht_device.humidity
f.write('{0},{1},{2:0.1f}*C,{2:0.1f}*F,{3:0.1f}%\r\n'.format(time.strftime('%m/%d/%y'), time.strftime('%H:%M'), temperature_c, temperature_f, humidity))
except RuntimeError as err:
print(err.args[0])
time.sleep(30)
Copy
4. Once done, save the file by pressing CTRL + X, then Y followed by ENTER.
5. Now like our last script lets go ahead and now run it by entering the following command.
python3 ~/dht22/humidity_logger.py
Copy
6. Once you exit out of the script, you will now be able to check out the “humidity.csv
” file to see all the results that you have logged over time.
If you run the following command, you should see the contents of the file.
nano ~/dht22/humidity.csv
Copy
7. Below is an example of the contents that you should see within this file.
Date,Time,Temperature,Humidity
05/04/19,05:47,22.3*C,50.6%
05/04/19,05:48,22.2*C,50.4%
05/04/19,05:48,22.2*C,50.6%
05/04/19,05:49,22.2*C,50.4%
05/04/19,05:49,22.2*C,50.4%
05/04/19,05:50,22.2*C,50.3%
05/04/19,05:50,22.2*C,50.3%
05/04/19,05:51,22.2*C,50.2%
Once you have verified that the file is successfully being written, then you are finally done with programming your Raspberry Pi humidity sensor.
If you need a waterproof temperature sensor, then be sure to check out our DS18B20 temperature sensor tutorial. It covers everything you need to know, and it works perfectly alongside this sensor.
I hope that everything has worked correctly for you and you’re not collecting the humidity and temperature without issues. If you want to leave some feedback, then please don’t hesitate to leave a comment below.
Hello,
Thanks for this tutorial!
The DHT22 can work from 3.3V to 6V. Why using the 3.3V and not the 5V ?
Thanks in advance for your explanaination.
Hi Cramy,
This choice purely comes from the GPIO pins of the Raspberry Pi. They are not 5v tolerant but are capable of handling 3.3V. If you were to use 5V yo uwould need to set up some level shifting circuitry to bring the signal back down to 3.3v.
Using 5v on the GPIO pins can fry the Pi’s processor.
Kind regards,
Emmet
If you want to run this code at startup using rc.local or systemd, you need to add the following syntax after the f.print statement:
By default, Python doesn’t flush data down to a file until it exits, which it’ll never do if it’s started at boot and running forever…
sudo pip3 install Adafruit_DHT
keeps telling me i cannot install it, as it cannot detect whether it is a pi or BBB. i am using a raspberry pi 3+.
Hi Eric,
Can you please try using the following command instead.
The changes here will pass an option to the package, bypassing its device detection and forcing the Raspberry Pi.
Cheers,
Emmet
It works really fine, thanks a lot.
One remark: using the AM2302 the introduction to the Python script should change from “Adafruit_DHT_AM2302” to “Adafruit_DHT.AM2302. With a full stop instead of the underscore.
“If you are following this guide but are using either the DHT11 or the AM2302 you can use either “Adafruit_DHT.DH11” or “Adafruit_DHT_AM2302” respectively.”
Hi Robert,
Thank you for pointing out this mistake, I have made a correction to the tutorial so it references the current name.
Cheers,
Emmet
Nice tutorial, taught me a lot in a short while, getting me on the right track, many thanks!
Hi.
i think theres a typo in this:
Adafruit_DHT_AM2302
It should be this:
Adafruit_DHT.AM2302
Btw great article i managed to set a test rpi very quickly with it.
Humidity.py works no problem, humidity logging appears to be working, csv file created but no data stored or readable.
Checked program more than once can’t find anything wrong or missing, any ideas?
REGARDS
Hi Bob,
I quickly tested this and it appears to be working fine for me.
Are you seeing the error “Failed to retrieve data from humidity sensor” appear at all when running the CSV version of the script?
Cheers,
Emmet
Hi Emmet
Thanks for reply. No data is present in csv file, when checked. Going to re-type code and see if this sorts out the hiccup. Could be I need to run update again in case file is corrupt. Watch this space for be cheer if successful.
Regards
Add an f. flush() command (to force flushing the data into the file) after the f.write(‘{0},{1},{2:0.1f}*C,{3:0.1f}%\r\n’.format(time.strftime(‘%m/%d/%y’), time.strftime(‘%H:%M’), temperature, humidity))
Thank you for the guide, it’s really easy to follow. Me and my son are learning together! Would it be possible to create a new CSV file everyday?
Yes, you can write the date into the csv name so it’s unique every day.
Something like this will get the date.
date = datetime.today().strftime('%Y-%m-%d')
Next, you will need to add it to your file name.
f = open('/home/pi/'+date+'humidity.csv', 'a+')
if os.stat('/home/pi/'+date+'humidity.csv').st_size == 0:
f.write('Date,Time,Temperature,Humidity\r\n')
I haven’t tested these blocks of code, but in theory it should work.
To add to Gus’s comment.
You would probably want to nest the code inside of a loop which first checks to see if the date has changed. An outline would be something like the following:
if the date is the same – run the code in the article with the changes shown in his comments.
Else – the date is different call `f.close` to close the current file.
when the code loops it will create a new file with the new date.
you also might want to consider changing the sleep time to something more reasonable like every 10 min instead of every 30s. 30s is nice for testing purposes, but for logging you’re going to end up with upwards of 2800 lines per day. Depending on what you’re checking you probably wont see much fluctuation between every 30s. say if you are checking your home’s temp for example.
My readings are so wonky any idea on this? it is a new sensor DHT11 , might it just be bad?
readings…
Temp=512.1*C Humidity=1612.8%
Hi Lisa,
It could be dodgy, a lose connection, or a mistake in the circuit.
Also, just want to check that you updated the code to reference DHT11 and not DHT22?
After replacing a DHT22 that had gone bad by a brandnew one I got values like you have. A 2nd new one produced reasonable values for a short time then showed faulty ones like yours… Any clues yet??
Hi Emmet,
pretty cool article, thanks!
Could I use a 4,7 kΩ resistor instead of a 10 kΩ one? (Because I have some…)
Will it works fine?
Thank you.
Hi Clo,
From memory you should be fine using a a 4.7k ohm resistors.
Cheers,
Emmet
Just a tip, the smaller the resistor on your pull-up (it pulls the signal level up from a weak output) the more current the part will have to sink. This could make it heat up, but I doubt it. It might, over time, cause electrical wear. If you’re trying to use battery power it will drain your battery faster, etc.
doing this seems so strait forward and then this………………..
python3 ~/humidity.py
File “/home/pi/humidity.py”, line 5
if humidity is not None and temperature is not None:
^
IndentationError: unindent does not match any outer indentation level
pi@raspberrypi:~ $
….chkl……. Thank you
p.s. and how would one modify or delete and retry?”
Hi sllimj,
Make sure that all of your code is properly indented as shown within the tutorial.
Python is sensitive to whitespace.
Cheers,
Emmet
Hello. New to electronics here, had a question about the resistor. Seeing that the DHT22 works with a voltage of 3.3 – 6V, why would you need the 10k resistor? Shouldn’t it safely work by itself?
Hi Gabriel,
In this case we aren’t using the resistor to bring down the voltage.
Instead we are using it to “pull-up” the input to HIGH to ensure that we have a defined valid logic level for when the pin is switching from input to output.
While you could exclude the resistor you will likely start to get unreliable measurements from the sensor.
Cheers,
Emmet
Hi so for some reason the “sudo apt-get install python3-dev python3-pip” command won’t work on my raspberry pi.
I tried to follow all the other steps in this tutorial but my raspberry pi powers off every time I run this code and I’m not sure why… Any recommendations? I have tried this on 2 different Raspberry Pis and it happens for both of them.
Thanks
Hi,
That does not sound right at all, make sure that you have a stable power supply to your Raspberry Pi and that the OS is not currupted.
There is no reason those install commands would cause a power off of your Raspberry Pi.
Cheers,
Emmet
This occurs when you have the positive and gnd pins reversed on the DHT; in this case the outer 2 pins, connected to pins 1 and 4, are reversed.
the python3 ~/humidity_logger.py command is taking a significant amount of time to run. Is this normal?
No, this isn’t normal. The script should respond immediately then every thirty seconds. Alter the time.sleep() value to read the sensor more often.
If the file creation fails, then this could be causing issues as well. Do you have any errors?
How do I convert C to Fahrenheit?
Add this line to the code.
Make sure you place it after this line.
Hello, How can I get 30 seconds between reading using the code.
Thank you for your help.
Hi,
To add a 30 second gap between readings you will need to import the time library and sleep the script for 30 seconds.
Add time with the other import.
At the end of the while loop add.
I hope that helps!
Cheers,
Gus
It seems the csv file only updates once the program is stopped. Is that’s correct? Or is there a way of updating the csv within the code?
Hi Stephen,
Can you try updating the following line.
f = open('/home/pi/humidity.csv', 'a+')
To
f = open('/home/pi/humidity.csv', 'a+', 0)
This should tell Python not to buffer writes so every write should be applied instantly to the file.
Alternatively you can
f.flush()
on every loop.Cheers,
Emmet
amt1001 I tried to connect this sensor, it does not work. Do you have experience connecting and working with it? on python
This tutorial is for digital sensors such as the DHT22, DHT11, and the AM2302. The amt1001 appears to be analog sensor so it will require a bit more work to get it working with the Raspberry Pi. You will likely need to use a analog to digital converter.
Hi,
I have got the sensor to return data in the terminal but it isn’t writing to the file although it has generated a csv. After a while it did return a failed to return data from the sensor error. Is there any reason why it would work with one script and not the other?
There shouldn’t be any reason why it would work in one but not the other. I will look into the error and see if I can recreate it locally.
hello
when I run the code an import error shows up “importError: No module named Adafruit_DHT”
This means the module isn’t installed. Have you run the install command? If so, did it error?