In this tutorial, I will be going through the steps on how to set up your very own Raspberry Pi temperature sensor.
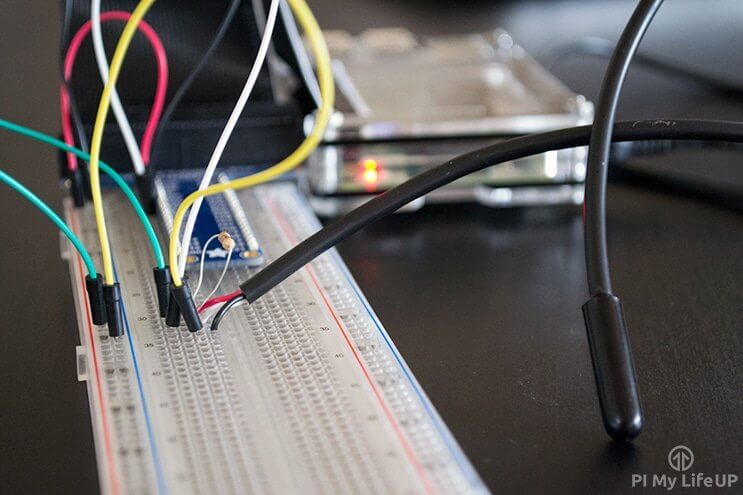
Like most of the sensor tutorials, the process of setting up this sensor is pretty straightforward and consists of a basic circuit and some code.
I will be using a ds18B20 waterproof sensor, and this probe can provide temperatures over a one-wire interface. Even better this is waterproof making it perfect if you need to use the sensor in a wet environment.
There are many other temperature sensors that you can use with your Raspberry Pi but this tutorial we will be focusing on the ds18b20.
You will find the ds18b20 temperature sensor handy in a lot of situations, especially when it comes to monitoring the temperatures of surfaces and liquids.
Equipment
The equipment that you will need for this Raspberry Pi temperature sensor is listed below.
Keep in mind the breadboard, and the breadboard wire is optional, but I highly recommend investing in these as they may make working with circuitry a lot easier.
Recommended
- Raspberry Pi Amazon
- Micro SD Card Amazon
- DS18B20 Temperature Sensor Amazon
- 4.7k ohm Resistor Amazon
- GPIO breakout kit Amazon
- Breadboard Amazon
- Breadboard Wire Amazon
Optional
Video
If you want to see how to put together the circuit and a rundown of the code, then be sure to check out my video below.
I go through everything there is to know about this cool little circuit in the video. You can also find the full written tutorial right under the video.
Building the Raspberry Pi ds18b20 Circuit
The circuit that we will need to build is pretty straightforward as we only need a resistor and the temperature sensor.
The sensor that I am using in this tutorial is a waterproof version of the DS18B20 sensor. It simply looks like a very long cord with a thick part on one end. If you have just a plain version without wiring and waterproofing, then it looks exactly like a transistor.
This sensor is pretty accurate being within 0.05°C of the actual temperature. It can handle temperatures of up to 125°C (260°F), but it’s recommended you keep it below 100°C (210°F). This device also has an onboard analog to digital converter so we’re able to easily hook it up to a digital GPIO pin on the Pi.
Keep in mind that not all temperature sensors are the same. Something like the TMP36 will not simply replace the ds18b20 in this tutorial.
The TMP36 is an analogue device making it slightly harder to integrate with the Pi. The TMP36 has basically the same problem we had with the light sensor, and this is because the Raspberry Pi doesn’t have any analogue pins.
Putting this circuit together is super simple, I will quickly go through some basic instructions below. If you’re having trouble following them, then please refer to the video or diagram.
If you need more information on the GPIO pins, then be sure to check out my guide to getting started with the Raspberry Pi GPIO pins.
1. First, connect the 3v3 pin from the Pi to the positive rail and a ground pin to the ground rail on the breadboard.
2. Now place the DS18B20 sensor onto the breadboard.
3. Place a 4.7k resistor between the positive lead and the output lead of the sensor.
4. Place a wire from the positive lead to the positive 3v3 rail.
5. Place a wire from the output lead back to pin #4 (Pin #7 if using physical numbering) of the Raspberry Pi.
6. Place a wire from the ground lead to the ground rail.
Once done, your circuit should look similar to the diagram below. Keep in mind some versions of the temperature sensor may have more wires than just three. Refer to the datasheet for more information for each of the wires.
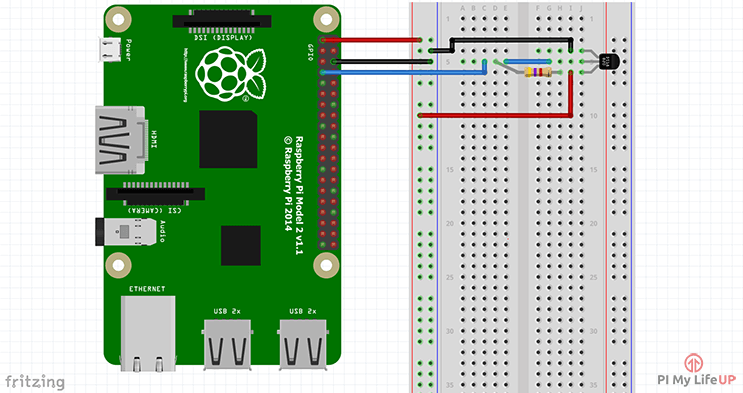
The Raspberry Pi Temperature Sensor Code
The code for setting up the temperature sensor is a little more complicated than the circuit itself. This complexity is just because of the way we need to handle the data that comes from the sensor.
As we’re using Python, it will be worth learning some of the basics, so I highly recommend checking out our Python beginner’s guide.
Before we make the Python script, we first need to setup the Pi so it can read data from the sensor. To do this, we need to add OneWire support.
1. To add support for OneWire, we first need to open up the boot config file, and this can be done by running the following command. I like to use the nano text editor.
sudo nano /boot/config.txt
Copy
2. At the bottom of this file enter the following.
dtoverlay=w1-gpio
Copy
3. Once done save and exit by pressing CTRL + X and then Y. Now reboot the Pi by running the following command.
sudo reboot
Copy
4. You can skip to downloading the code onto the Pi or follow the next few steps to check that the sensor is actually working.
5. Once the Raspberry Pi has booted back up, we need to run modprobe
so we can load the correct modules.
sudo modprobe w1-gpio
sudo modprobe w1-therm
Copy
6. Now change into the devices directory and use the ls command to see the folders and files in the directory.
cd /sys/bus/w1/devices
ls
Copy
7. Now run the following command, change the numbering after cd
to what has appeared in your directory by using the ls
command. (If you have multiple sensors there will be more than one directory)
cd 28-000007602ffa
Copy
8. Now run the following cat command.
cat w1_slave
Copy
9. This command should output data but as you will notice it is not very user-friendly.
The first line should have a YES or NO at the end of it. If it is YES, then a second line with the temperature should appear. This line will look similar to something like t=12323, and you will need to do a bit of math to make this a usable temperature that we can understand easily. For example, Celsius you simply divide by 1000.
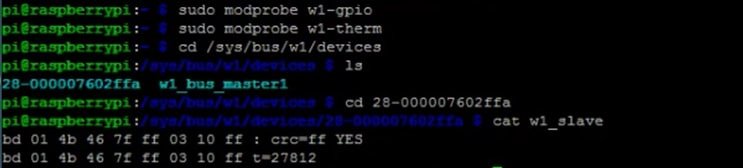
Now it’s time to move onto the Python script.
I’ll briefly explain the code below if you want to learn more about it. This code was sourced from the tutorial over at Adafruit. If you want to download it, you can simply download it at the Pi My Life Up GIT page or by using the following command.
git clone https://github.com/pimylifeup/temperature_sensor.git
Copy
To begin the Python script, we import three packages, OS, Glob and time.
Next, we run the modprobe commands, and these commands are the same as what we used before.
We declare three different variables that will point to the location of our sensor data. You shouldn’t have to change any of these.
import os
import glob
import time
os.system('modprobe w1-gpio')
os.system('modprobe w1-therm')
base_dir = '/sys/bus/w1/devices/'
device_folder = glob.glob(base_dir + '28*')[0]
device_file = device_folder + '/w1_slave'
Copy
In the read_temp_raw
function we open up the file that contains our temperature output. We read all the lines from this and then return it so the code that has called this function can use it. In this case the read_temp
function calls this function.
def read_temp_raw():
f = open(device_file, 'r')
lines = f.readlines()
f.close()
return lines
Copy
In the read_temp
function we process the data from the read_temp_raw
function. We first make sure that the first line contains YES. This means there will be a line with a temperature in it.
If there is a temperature we then find the line with t=
by using lines[1].find('t=')
. Lines[1] means we’re looking at the 2nd element in the array, in this case, the 2nd line.
Once we have the line we simply get all the numbers that are after the t=
and this is done at lines[1][equals_pos+2:]
. Equals_pos
is the start position of the temperature (t), and we add 2 to the position, so we only get the actual temperature numbers.
We then convert the number into both a Celsius and Fahrenheit temperature. We return both of these to the code that called this function. This function is the print located in the while loop.
def read_temp():
lines = read_temp_raw()
while lines[0].strip()[-3:] != 'YES':
time.sleep(0.2)
lines = read_temp_raw()
equals_pos = lines[1].find('t=')
if equals_pos != -1:
temp_string = lines[1][equals_pos+2:]
temp_c = float(temp_string) / 1000.0
temp_f = temp_c * 9.0 / 5.0 + 32.0
return temp_c, temp_f
Copy
The while loop is always true so it will run forever until the program is interrupted by an error or by the user cancelling the script. It simply calls the read_temp
within the print function. The print function allows us to see the output on our screen. The script is then put to sleep for 1 second every time it has read the sensor.
while True:
print(read_temp())
time.sleep(1)
Copy
Testing the Code
Once you have either downloaded or finished writing up the code and you also have set up the circuit correctly, we can now call the Python script. To call the Python script simply run the following command.
sudo python thermometer_sensor.py
Copy
You should now have an output of temperatures in both Fahrenheit and Celsius. You can alter this just to display your preferred temperature scale.
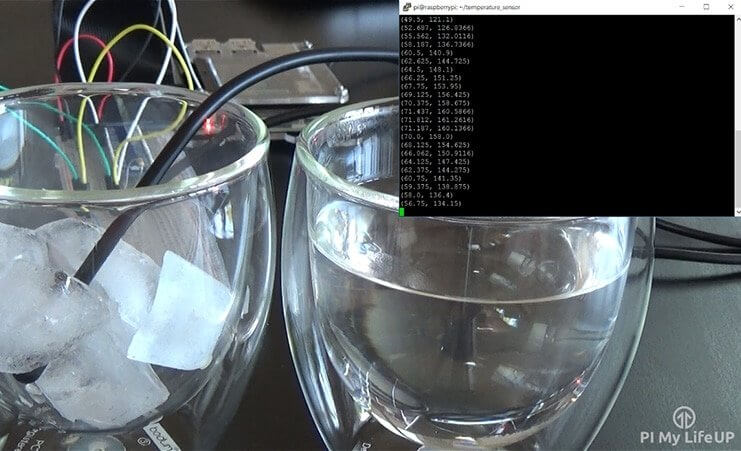
That’s everything you need to know for getting your Raspberry Pi ds18b20 temperature sensor up and running.
If you’re looking to extend this one step further, then be sure to check out my tips below. I will be looking at incorporating this sensor into future projects so stay tuned.
Possible Further Work
There are quite a few more things you’re able to do with this Raspberry Pi temperature sensor. I will quickly mention a couple of ideas, and some of them I may cover in the future.
You can have the Python script connect to a database such as MYSQL and store the data that way. If you add a timestamp to the data, you will be able to look back on data in the future and compare any changes.
This next tip would work great with the one above. You can use the data stored in the MYSQL database to make nice pretty graphs to show to temperature over the course of a day, month or even year. You could make it plot a graph in real time too.
Alternatively, using influxDB along with Grafana is a great way of visually displaying your temperature statistics.
I hope you have been able to build and get this Raspberry Pi temperature sensor working.
If you have come across any problems, have feedback or anything else then please feel free to leave a comment below.
Danke für die Mega Anleitung, hat geklappt 🙂
Admin Edit: [Google English Translation]: Thanks for the mega instructions, it worked 🙂