In this Raspberry Pi accelerometer project, we will show you how to connect the ADXL345 accelerometer to your Raspberry Pi. We also show how to program a Python script to interact with it.
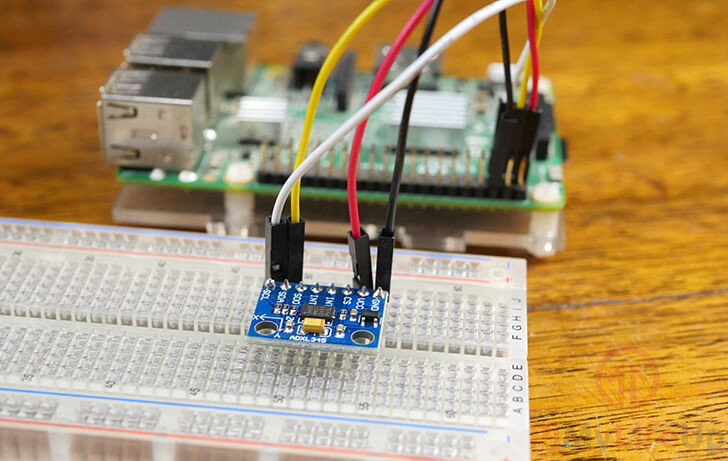
This tutorial will walk you through the process of wiring the ADXL345 to your Raspberry Pi. It also goes through the steps on how to implement Adafruit’s ADXL34x library to talk with the device. Both are relatively simple processes.
The ADXL345 is a low power, 3-axis (It tracks both X, Y and Z accelerations) MEMS accelerometer that utilizes the I2C and SPI serial interfaces.
For those who do not know, MEMS stands for Micro Electro Mechanical System. In the case of the accelerometer, this means that it has a microscopic component that is utilized to calculate the current acceleration being felt by the sensor.
The support of both I2C and SPI serial protocols makes it incredibly easy to use with our Raspberry Pi as we don’t require any additional hardware to interpret the data coming from the accelerometer.
There are plenty of other sensors for the Raspberry Pi that will work great with this accelerometer. For example, a distance sensor will be great at measuring how the sensor is to objects.
Equipment
Below is all the equipment that you will need for setting up the ADXL345 accelerometer with your Raspberry Pi.
Recommended
- Raspberry Pi Amazon
- Micro SD Card Amazon (8GB+ Recommended)
- Power Supply Amazon
- Breadboard wire Amazon
- ADXL345 Accelerometer Amazon
Optional
Video
In this video, we walk you through the process of connecting the ADXL345 Accelerometer to the Raspberry Pi. We also show you how to set up your Raspberry Pi to talk with the device.
If you prefer a more thorough written guide, then you can continue below.
Raspberry Pi ADXL345 Accelerometer Setup
In this part of the tutorial, we will be showing you how you can connect the digital ADXL345 accelerometer to the Raspberry Pi.
As the ADXL345 is a digital sensor, you won’t have to deal with any additional circuitry such as an analog to digital converter. Being digital means. you can wire the sensor directly to the Raspberry Pi.
Follow our guide below to see how to set up the Raspberry Pi with the ADXL345 accelerometer.
ADXL345 Accelerometer Circuit
Below we have included two ways of showing you how to connect the accelerometer to your Raspberry Pi.
You can either follow the diagrams or utilize our written steps on the wires and the GPIO pins that you need to use.
- Wire the GND pin of the Accelerometer to Physical Pin 6 (GND) on the Raspberry Pi.
- Wire the VCC pin of the Accelerometer to Physical Pin 1 (3v3) on the Raspberry Pi.
- Wire the SDA pin of the Accelerometer to Physical Pin 3 (SDA) on the Raspberry Pi.
- Wire the SCL pin of the Accelerometer to Physical Pin 5 (SCL) on the Raspberry Pi.
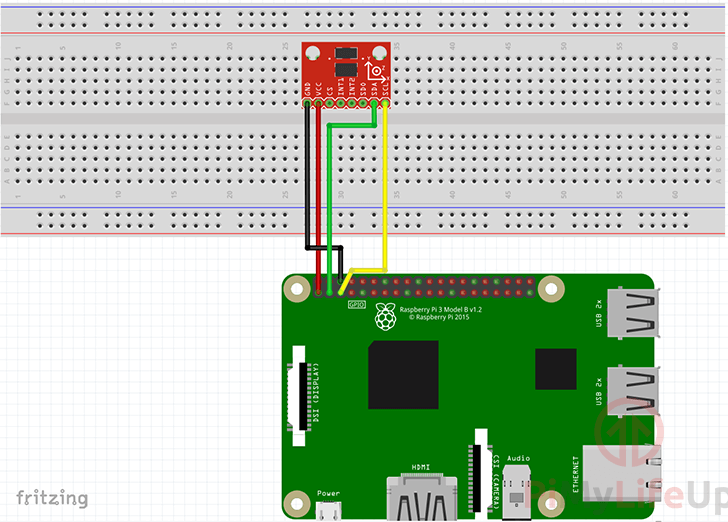
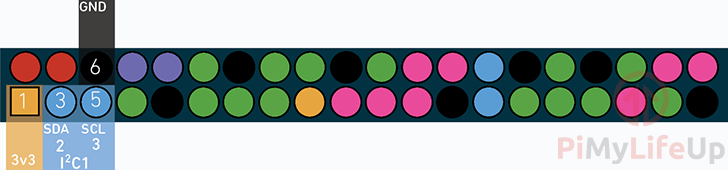
Preparing your Raspberry Pi to Talk with the Accelerometer
1. Before we can get our Raspberry Pi to retrieve data from our ADXL345 Accelerometer, there are a few changes we must make to the Pi’s configuration.
Let’s first ensure that everything is up to date by running the following two commands.
sudo apt-get update
sudo apt-get upgrade
Copy
2. Once the Raspberry Pi has finished updating, we will need to go ahead and launch the Raspberry configuration tool so that we can enable I2C on the Raspberry Pi.
Run the following command to launch the raspi configuration tool.
sudo raspi-config
Copy
3. On this screen, you need to head to the “5 Interfacing Options” menu.
You can navigate the raspi-config tools menus by using the ARROW keys. Use the ENTER key to select particular options.
4. Now within the interfacing options menu go ahead and select “P5 I2C“.
When asked if you would like to enable the ARM I2C interface, select “<YES>“.
5. After enabling the I2C interface, you will need to restart your Raspberry Pi by running the following command.
sudo reboot
Copy
6. Now that we have enabled I2C and restarted the Raspberry Pi, we can now proceed to install the packages that we will rely on to talk with our accelerometer.
Run the following command to install.
sudo apt-get install python3-dev python3-pip python3-smbus i2c-tools -y
Copy
6. With all our required packages installed let’s now check to see whether our Raspberry Pi can see our ADXL345 Accelerometer.
We can do that by running the following command.
sudo i2cdetect -y 1
Copy
From this command, you should see a fair bit displayed on the command line. Within this result, you should at least see a number such as “53
“.
If nothing appears then make sure you have connected your ADXL345 Accelerometer to the Raspberry Pi correctly and that all solder points on the pins of the sensor are clean. If you see an error try re-enabling I2C again.
Coding a Script to Talk with the ADXL345 Accelerometer
1. With all the packages that we need now installed to the Raspberry Pi, we can now proceed to write a small Python script. This script is so that we can read information from the accelerometer.
To talk with the ADXL345, we will be making use of Adafruit’s ADXL34x Python library. To install this library so that we can utilize it, you need to run the following pip
command.
sudo pip3 install adafruit-circuitpython-ADXL34x
Copy
2. With the library installed, we can now proceed to code our small Python script.
Begin writing this file by running the following command.
nano ~/accelerometer.py
Copy
3. Within our new script, write the following lines of code.
import time
Copy
We start by importing the “time
” library. We utilize the “time
” library so that we can put the script to sleep for a short period.
import board
Copy
Here we import Adafruit’s “board
” library. This unique library is designed to quickly know what pins are available on a device.
So instead of specifying a specific pin, we can use something like “board.scl
” and that will return the correct pin for the current microcontroller. In our case, this is the Raspberry Pi.
import busio
Copy
Next, we import Adafruit’s “busio
” module. This module contains a variety of different libraries to handle various serial protocols. We will be using this modules library for handling the I2C serial protocol.
import adafruit_adxl34x
Copy
Now we import the “adafruit_adxl34x
” library. This library contains all the code we need for reading information from our ADXL345 accelerometer and makes the process incredibly simple.
i2c = busio.I2C(board.SCL, board.SDA)
Copy
Here we utilize the “busio
” library to prepare an I2C connection for our current boards SCL and SDA pins. We store the handle into our “i2c
” variable.
accelerometer = adafruit_adxl34x.ADXL345(i2c)
Copy
We now instantiate the ADXL345 library into our “accelerometer
” object. We will utilize this object to read and obtain information from our sensor. Into the constructor for the library, we pass in our I2C handle.
while True:
print("%f %f %f"%accelerometer.acceleration)
time.sleep(0.5)
Copy
Here we start an infinite loop by using “while True:
“.
Within this infinite loop, we print out the X, Y, and Z acceleration values that have been retrieved from the accelerometer by the library.
Once we have printed the X, Y, and Z values, we then put the script to sleep for half a second.
We sleep the script to stop it from flooding the command line with the values provided by the accelerometer.
4. The final version of the accelerometer code should end up looking like what we have below. Check your final code against this.
import time
import board
import busio
import adafruit_adxl34x
i2c = busio.I2C(board.SCL, board.SDA)
accelerometer = adafruit_adxl34x.ADXL345(i2c)
while True:
print("%f %f %f"%accelerometer.acceleration)
time.sleep(1)
Copy
When you are happy that all the code is correct you can save it by pressing CTRL + X then Y followed by ENTER.
5. With the code now done, let’s go ahead and run the script by running the following command.
python3 ~/accelerometer.py
Copy
6. By running this code, you should start seeing the values being read in from the accelerometer.
0.117680 0.313813 8.355266
0.117680 0.313813 8.394492
0.117680 0.313813 8.355266
0.117680 0.353039 8.316039
0.117680 0.431493 8.316039
0.117680 0.313813 8.276813
7. Now that we know that the accelerometer is returning data and that the Raspberry Pi is reading correctly, we can explore some of the other functionality of the ADXL345 library.
Exploring the ADXL345 Accelerometer Library
1. In this section of this Raspberry Pi accelerometer tutorial, we will be exploring some of the other functionality provided by Adafruit’s ADXL345 accelerometer library.
In particular, we will be looking at the “events
” that the library can automatically detect when enabled.
To begin, let’s open our “accelerometer.py
” script by using the following command.
nano ~/accelerometer.py
Copy
2. Within the file, find and add the lines shown below. We will explain what each new section is for and what it does.
Find
accelerometer = adafruit_adxl34x.ADXL345(i2c)
Copy
Add the following lines below the line above.
accelerometer.enable_freefall_detection(threshold=10, time=25)
Copy
This line enables the libraries freefall detection event. It takes in two variables, one being the threshold, the other is the time.
The threshold
is the value that acceleration on all axes must be under for it to register as a drop. The scale factor is 62.5 mg, so our example is 10*62.5=625 mg.
Next, the time
variable is the amount of time that acceleration on all axes must be less than the threshold for them to register as being dropped. The scale factor is 5 ms, so our example is 25×5=125ms.
accelerometer.enable_motion_detection(threshold=18)
Copy
This line enables the libraries motion detection event. It takes in one variable, the threshold.
The threshold
variable is the value that acceleration on all axes must exceed for a motion to be detected. If you find this is too sensitive or not sensitive enough you can modify this value. The scale factor is 62.5 mg, so our example is 18*62.5=1125 mg.
accelerometer.enable_tap_detection(tap_count=1, threshold=20, duration=50, latency=20, window=255)
Copy
This line enables the libraries tap detection event.
The tap_count
variable is whether you want to detect a single tap or a double tap. Set this to 1 for single tap or 2 for double taps.
Next is the threshold
variable, and this is for how sensitive you want the tap to be, the higher the value, the less sensitive the code will be to detecting a tap. Like the other threshold variables the scale factor is 62.5 mg.
The duration
variable is the length of time in nanoseconds that the motion should occur. If the duration of the movement is too long, it will not be detected as a tap. This value needs to be in ms.
Now the latency
variable is the length of time after the initial impulse to start looking for the second tap. The latency value should be in ms.
Lastly, the window
variable is the length of time in which the code should look for the second tap to occur. Again, this value should be in ms.
Find
print("%f %f %f"%accelerometer.acceleration)
Copy
Add the following lines below
print("Dropped: %s"%accelerometer.events["freefall"])
print("Tapped: %s"%accelerometer.events['tap'])
print("Motion detected: %s"%accelerometer.events['motion'])
Copy
These additional lines print out the status of all the events that we activated earlier in the script. These print lines will let you know whether any of the events are currently being triggered.
Typically you won’t be checking for all these events at one time.
3. With all the changes made to the accelerometer code, it should look a bit like what we have below.
import time
import board
import busio
import adafruit_adxl34x
i2c = busio.I2C(board.SCL, board.SDA)
accelerometer = adafruit_adxl34x.ADXL345(i2c)
accelerometer.enable_freefall_detection(threshold=10, time=25)
accelerometer.enable_motion_detection(threshold=18)
accelerometer.enable_tap_detection(tap_count=1, threshold=20, duration=50, latency=20, window=255)
while True:
print("%f %f %f"%accelerometer.acceleration)
print("Dropped: %s"%accelerometer.events["freefall"])
print("Tapped: %s"%accelerometer.events['tap'])
print("Motion detected: %s"%accelerometer.events['motion'])
time.sleep(0.5)
Copy
When you are happy all the code is correct you can save it by pressing CTRL + X then Y followed by ENTER.
4. With the code now done, let’s go ahead and run the script by running the following command.
python3 ~/accelerometer.py
Copy
5. By running this code, you should start seeing text be printed to the command line, showing the X, Y, and Z positions received as well as the status of all the events.
0.235360 0.353039 8.355266
Dropped: False
Tapped: False
Motion detected: False
With that all done, you should now have some understanding of how to use your ADXL345 accelerometer with the Raspberry Pi.
I hope that this Raspberry Pi accelerometer tutorial has been able to teach you all the basics to setting up the ADXL345. If you have any questions, feedback, or anything else, then please don’t hesitate to leave a comment below.
Need faster help? Premium members get priority responses to their comments.
Upgrade for Priority Support