In this tutorial, I will be going through the steps on how to set up the Arduino serial monitor so you can debug and interact with a program running on the Arduino.
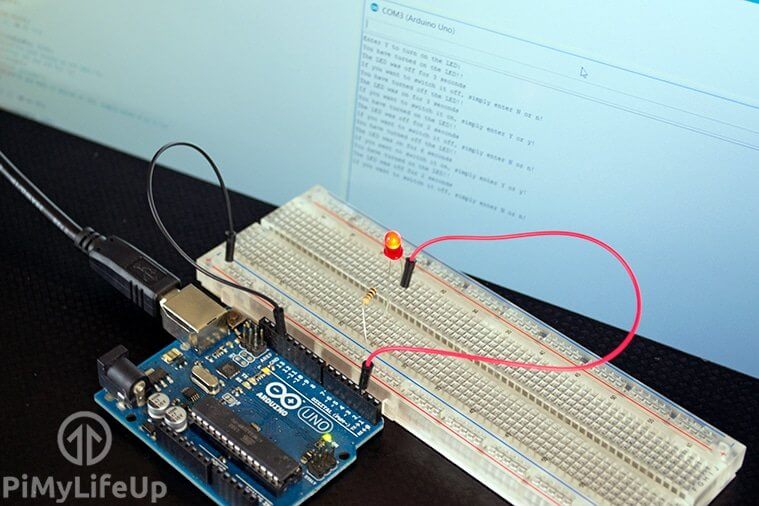
This tutorial is pretty simple, but it can be a bit confusing at first especially if you’re new to programming and the Arduino.
As part of this tutorial, we will build a simple circuit to demonstrate how you can both receive and send commands over the serial monitor.
Learning how to use the serial monitor is important as you will need to use it to debug your code and circuits.
Equipment
The equipment that I use in this simple Arduino serial monitor is listed below.
Recommended
Optional
You can easily learn everything without using the example circuit but I find doing is often the best way to understand how things work.
Video
If you want to see my video on the process of setting up the serial monitor, then be sure to check it out below.
I go through all the steps to set up the circuit, code and also being able to open and use the monitor window correctly.
You can check out the full written guide right underneath the video.
The Circuit for the Example
The circuit that we will be building for this tutorial is super simple and will consist of an LED that we will be able to control from the serial monitor. This is purely just there to show both sending and receiving of data through the monitor.
To set up the circuit simply do the following. If you’re having trouble, please refer to the circuit diagram.
1. Simply connect the positive lead of the red LED up to pin 2.
2. Next, hook a 100-ohm resistor from the negative lead to the ground rail on the Arduino.
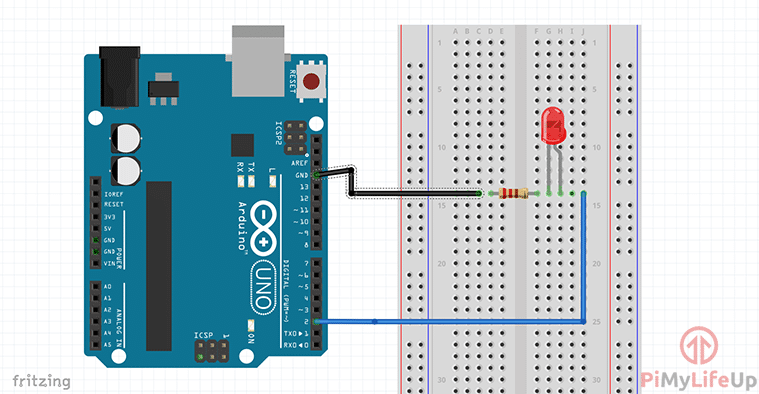
The Arduino Serial Monitor Code
The code that we will be using is simple, its purpose is to show the basics of how you can use the Arduino Serial monitor to view and send data.
I will explain each of the lines of code that we’re using and how you can use it in your next project to debug or interact with the Arduino.
If you just want to download the code straight away, then you can download it from our download mirror or on our GitHub.
To begin, we initialize an integer variable for our LED, this is the pin number leading to the LED. We also initialize a variable called count, this will store the amount of time the LED is on or off.
int redLedPin = 2;
int count = 0;
Copy
In the setup function, we first set the red LED to act as an output so we can turn it off and on.
We next call the serial.begin
function with a parameter value of 9600. The value we’re setting is known as the bits per second (baud) rate.
The baud rate can be set to quite a few different rates, but we will use 9600 as this seems to be the default and will work with most devices without any issues. It is very important to call this function. Otherwise you won’t be able to communicate with the Arduino.
Next, we wait for the serial interface to initialize and connect to the computer. We then call a function called Serial.println(). This function allows us to print a line to the Arduino serial monitor. You will notice this will print the text and then start on a new line for the next input or output.
void setup() {
pinMode(redLedPin, OUTPUT);
Serial.begin(9600);
while (! Serial);
Serial.println("Enter Y to turn on the LED:");
}
Copy
Lastly, we have the loop, this will continually loop through until either a new program is uploaded or the Arduino is switched off.
Inside the loop function, we have an if statement that is checking to see if there is data waiting in the serial buffer; “Serial.available
“. In simple terms, we’re checking to see if we have sent a command to it. If there is data, we enter the if statement.
Next, we create a variable called “ch
” and call “Serial.read()
” which will get us the data currently waiting in the serial buffer. Keep in mind this function will only get us the first byte of the data that is incoming to the Arduino. If you want to get an entire string you will need to use something like “Serial.readString
“.
If the byte of data is either y
or n
, we then enter the relevant if statement. In here you will notice we change the output of the LED pin high or low. We then print out a series of statements.
There are two things that you should notice here. “Serial.println
” will print the data and go to a new line while “Serial.print
” will print the data but stay on the same line when outputting.
You will also notice you can print variables such as the count example in the code below. Doing this will allow you to be able to debug values when it comes to data processing.
Lastly, I delay by a second and add 1 to count. This code is just showing you an example of adding data to a variable then printing it via the serial output.
void loop(){
if (Serial.available()){
char ch = Serial.read();
if (ch == 'y' || ch == 'Y'){
digitalWrite(redLedPin, HIGH);
Serial.println("You have turned on the LED!!");
Serial.print("The LED was off for ");
Serial.print(count);
Serial.println(" seconds");
Serial.println("If you want to switch it off, simply enter N or n!");
count = 0;
}
if (ch == 'n' || ch == 'N'){
digitalWrite(redLedPin, LOW);
Serial.println("You have turned off the LED!!");
Serial.print("The LED was on for ");
Serial.print(count);
Serial.println(" seconds");
Serial.println("If you want to switch it on, simply enter Y or y!");
count = 0;
}
}
delay(1000);
count += 1;
}
Copy
Once you’re done, you should be able to deploy the code onto the Arduino. In the next part, I will show you how to connect, receive and send data to the Arduino.
Connecting, Receiving and Sending Data to the Arduino
Now that the code has been uploaded to the Arduino we will need to open up the Arduino serial monitor. This is pretty easy, and there are only a few options that I will need to explain.
To open up the serial monitor, go up to tools and then select the serial monitor. Alternatively, CTRL + SHIFT + M will also bring up the same window. Make sure you are connected to the Arduino otherwise the window won’t open up.
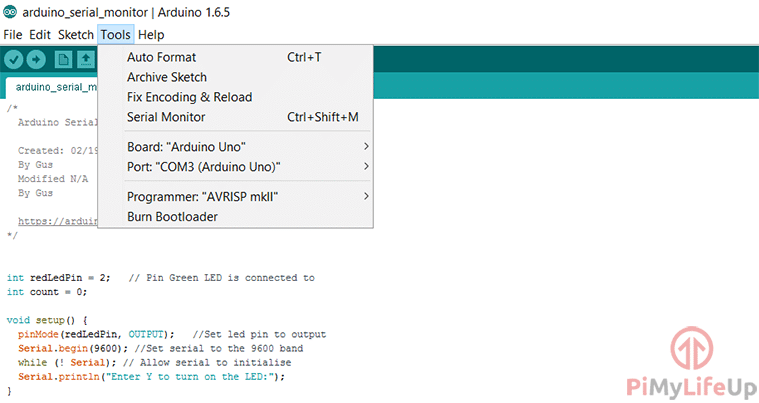
You should now have a window open that looks similar to the one below.
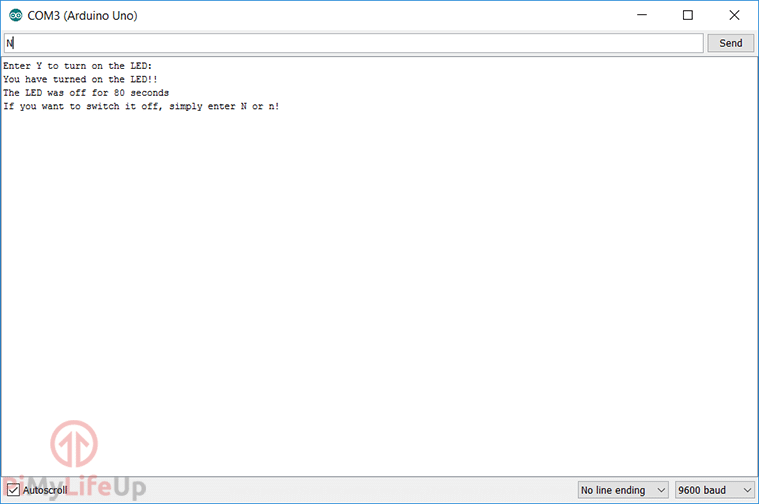
At the top of this screen, there should be an input area. This is where you can type and send a text to the Arduino. To send the text that you have typed simply press the send button.
Below this is the output window, all the data that is sent to us will be displayed here. If you’re using the example code above you will be able to see the text that is in the “Serial.println
” functions.
At the bottom of the screen, we have three different options.
- The first is pretty self-explanatory, the auto-scroll will disable and enable the automatically scrolling of the output box.
- Second, we have the line ending option. You can set the monitor to automatically append a line ending after what you enter/send to the Arduino.
- Thirdly we have the baud rate that I mentioned above. Make sure this matches to what you have set in the code. If it is different than the output text will appear as gibberish. If you’re receiving nonsense, then this is a likely cause of this.
Conclusion
This is basically everything you need to know to understand the basics of the serial monitor. There are a few more things you can learn about such as using strings via the serial interface and more but this probably all you need to know for now.
I hope you now understand how to use the Arduino serial monitor correctly and will help you with future Arduino projects. If you want to add anything to this tutorial, then be sure to leave a comment below.
Thank you! Great tutorial, and very practical examples.
One of the best most clear descriptions of how to use the monitor!
Possibly add a section on how to properly represent the displayed data to be readable in the desired format. Such as how to display numbers in decimal, hex, or binary. I think it now defaults to binary sometimes and decimal others.
Thank you for your feedback Ronna, that sounds like something we should include. Hopefully we will have included in the near future.
Thank you for this great tutorial.
Very helpful.
Just what I needed 🙂